Python: Frequency of the tuples in a given list
Write a Python program to get the frequency of the tuples in a given list.
Sample Solution:
Python Code:
# Import the Counter class from the collections module
from collections import Counter
# Create a list of tuples 'nums' with inner lists of pairs
nums = [(['1', '4'], ['4', '1'], ['3', '4'], ['2', '7'], ['6', '8'], ['5', '8'], ['6', '8'], ['5', '7'], ['2', '7'])]
# Print a message to indicate the display of the original list of tuples
print("Original list of tuples:")
# Print the content of 'nums'
print(nums)
# Use Counter to count the frequency of tuples after sorting them
result = Counter(tuple(sorted(i)) for i in nums[0])
# Print a message to indicate the display of tuples and their frequency
print("\nTuples", " ", "frequency")
# Loop through the results and print each tuple and its frequency
for key, val in result.items():
print(key, " ", val)
Sample Output:
Original list of tuples: [(['1', '4'], ['4', '1'], ['3', '4'], ['2', '7'], ['6', '8'], ['5', '8'], ['6', '8'], ['5', '7'], ['2', '7'])] Tuples frequency ('1', '4') 2 ('3', '4') 1 ('2', '7') 2 ('6', '8') 2 ('5', '8') 1 ('5', '7') 1
Flowchart:
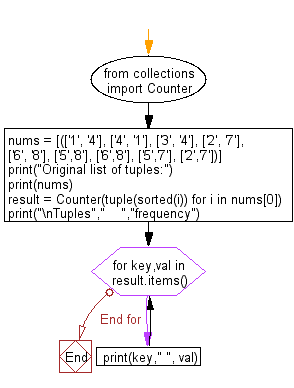
Python Code Editor:
Previous: Write a Python program to insert an element at the beginning of a given OrderedDictionary.
Next: Write a Python program to calculate the maximum aggregate from the list of tuples (pairs).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics