Python: Remove duplicate words from a given string use collections module
Python Collections: Exercise-27 with Solution
Write a Python program to remove duplicate words from a given string. Use the collections module.
Sample Solution:
Python Code:
# Import the OrderedDict class from the collections module
from collections import OrderedDict
# Create a string 'text_str'
text_str = "Python Exercises Practice Solution Exercises"
# Print a message to indicate the display of the original string
print("Original String:")
# Print the content of 'text_str'
print(text_str)
# Print a message to indicate the display after removing duplicate words from the string
print("\nAfter removing duplicate words from the said string:")
# Split 'text_str' into words, use OrderedDict to remove duplicates, and join the unique words
result = ' '.join(OrderedDict((w, w) for w in text_str.split()).keys())
# Print the result
print(result)
Sample Output:
Original String: Python Exercises Practice Solution Exercises After removing duplicate words from the said string: Python Exercises Practice Solution
Flowchart:
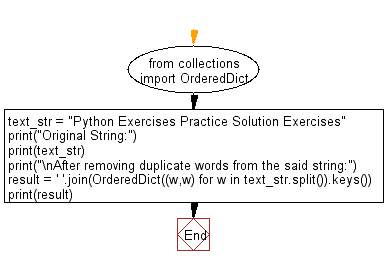
Python Code Editor:
Previous: Write a Python program to find the difference between two list including duplicate elements. Use collections module.
Next: Write a Python program to create a dictionary grouping a sequence of key-value pairs into a dictionary of lists. Use collections module.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/collections/python-collections-exercise-27.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics