Python: Grouping a sequence of key-value pairs into a dictionary of lists using collections module
28. Group key-value pairs into a dictionary using collections
Write a Python program to create a dictionary grouping a sequence of key-value pairs into a dictionary of lists. Use the collections module.
Sample Solution:
Python Code:
# Import the defaultdict class from the collections module
from collections import defaultdict
# Define a function 'grouping_dictionary' that groups a sequence of key-value pairs into a dictionary of lists
def grouping_dictionary(l):
# Create a defaultdict 'd' to store lists of values associated with each key
d = defaultdict(list)
# Loop through the key-value pairs in the input list 'l'
for k, v in l:
# Append the value 'v' to the list associated with key 'k' in 'd'
d[k].append(v)
# Return the resulting dictionary
return d
# Create a list of key-value pairs 'colors'
colors = [('yellow', 1), ('blue', 2), ('yellow', 3), ('blue', 4), ('red', 1)]
# Print a message to indicate the display of the original list
print("Original list:")
# Print the content of 'colors'
print(colors)
# Print a message to indicate the display after grouping the key-value pairs into a dictionary of lists
print("\nGrouping a sequence of key-value pairs into a dictionary of lists:")
# Call the 'grouping_dictionary' function with 'colors' and print the result
print(grouping_dictionary(colors))
Sample Output:
Original list: [('yellow', 1), ('blue', 2), ('yellow', 3), ('blue', 4), ('red', 1)] Grouping a sequence of key-value pairs into a dictionary of lists: defaultdict(<class 'list'>, {'yellow': [1, 3], 'blue': [2, 4], 'red': [1]})
Flowchart:
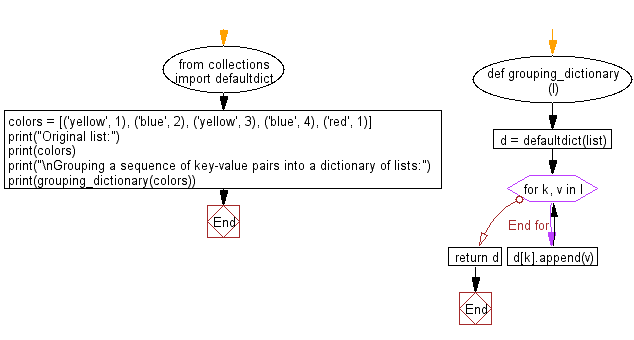
For more Practice: Solve these Related Problems:
- Write a Python program to group a list of tuples into a dictionary where each key maps to a list of values using collections.defaultdict.
- Write a Python program to iterate over a sequence of key-value pairs and build a dictionary of lists manually.
- Write a Python program to use dictionary comprehension and defaultdict to group items by their keys.
- Write a Python program to implement a function that accepts a list of tuples and returns a grouped dictionary of lists.
Go to:
Previous: Write a Python program to remove duplicate words from a given string use collections module.
Next: Write a Python program to get the frequency of the elements in a given list of lists. Use collections module.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.