Python: Count the most common words in a dictionary
Write a Python program to count the most common words in a dictionary.
Sample Solution:
Python Code:
# Create a list 'words' containing various color names and other words
words = [
'red', 'green', 'black', 'pink', 'black', 'white', 'black', 'eyes',
'white', 'black', 'orange', 'pink', 'pink', 'red', 'red', 'white', 'orange',
'white', "black", 'pink', 'green', 'green', 'pink', 'green', 'pink',
'white', 'orange', "orange", 'red'
]
# Import the Counter class from the collections module
from collections import Counter
# Create a Counter object 'word_counts' to count the occurrences of words in 'words'
word_counts = Counter(words)
# Find the four most common words using 'most_common' and store them in 'top_four'
top_four = word_counts.most_common(4)
# Print the four most common words
print(top_four)
Sample Output:
[('pink', 6), ('black', 5), ('white', 5), ('red', 4)]
Flowchart:
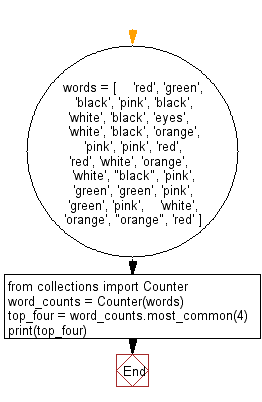
Python Code Editor:
Previous: Write a Python program to count the occurrence of each element of a given list.
Next: Write a Python program to find the class wise roll number from a tuple-of-tuples.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics