Python: Find the class wise roll number from a tuple-of-tuples
32. Find Class Wise Roll Number from a Tuple-of-Tuples
Write a Python program to find the class wise roll number from a tuple-of-tuples.
Sample Solution:
Python Code:
# Import the defaultdict class from the collections module
from collections import defaultdict
# Create a tuple 'classes' containing class names and roll numbers
classes = (
('V', 1),
('VI', 1),
('V', 2),
('VI', 2),
('VI', 3),
('VII', 1),
)
# Create a defaultdict 'class_rollno' to store lists of roll numbers for each class
class_rollno = defaultdict(list)
# Loop through the tuples in 'classes'
for class_name, roll_id in classes:
# Append the roll number 'roll_id' to the list associated with class 'class_name' in 'class_rollno'
class_rollno[class_name].append(roll_id)
# Print the resulting dictionary 'class_rollno'
print(class_rollno)
Sample Output:
defaultdict(<class 'list'>, {'V': [1, 2], 'VI': [1, 2, 3], 'VII': [1]})
Flowchart:
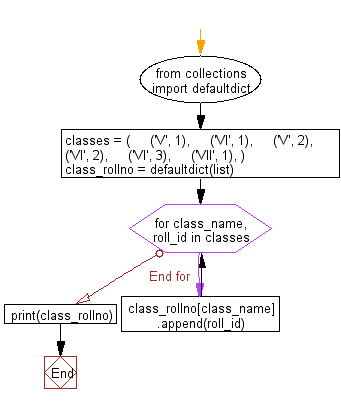
For more Practice: Solve these Related Problems:
- Write a Python program to group roll numbers by class from a tuple of tuples using collections.defaultdict.
- Write a Python program to iterate over a tuple of tuples and construct a dictionary where each key is a class and its value is a list of roll numbers.
- Write a Python program to use list comprehension to transform a tuple-of-tuples into a dictionary grouping student roll numbers by class.
- Write a Python program to implement a function that returns a grouped dictionary from a tuple-of-tuples containing class and roll numbers.
Go to:
Previous: Write a Python program to count the most common words in a dictionary.
Next: Write a Python program to count the number of students of individual class.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.