Python: Count the number of students of individual class
Python Collections: Exercise-33 with Solution
Write a Python program to count the number of students in an individual class.
Sample Solution:
Python Code:
# Import the Counter class from the collections module
from collections import Counter
# Create a tuple 'classes' containing class names and the number of students in each class
classes = (
('V', 1),
('VI', 1),
('V', 2),
('VI', 2),
('VI', 3),
('VII', 1),
)
# Create a Counter object 'students' to count the occurrences of class names in 'classes'
students = Counter(class_name for class_name, no_students in classes)
# Print the 'students' Counter object, which represents the count of students in each class
print(students)
Sample Output:
Counter({'VI': 3, 'V': 2, 'VII': 1})
Flowchart:
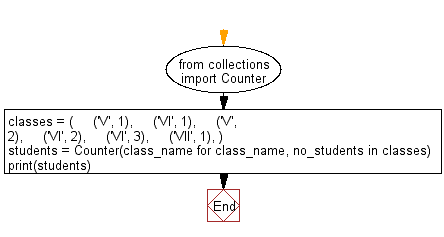
Python Code Editor:
Previous: Write a Python program to find the class wise roll number from a tuple-of-tuples.
Next: Write a Python program to create an instance of an OrderedDict using a given dictionary. Sort the dictionary during the creation and print the members of the dictionary in reverse order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/collections/python-collections-exercise-33.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics