Python: Group a sequence of key-value pairs into a dictionary of lists
Write a Python program to group a sequence of key-value pairs into a dictionary of lists.
Sample Solution:
Python Code:
# Import the defaultdict class from the collections module
from collections import defaultdict
# Create a list 'class_roll' containing tuples with class names and roll numbers
class_roll = [('v', 1), ('vi', 2), ('v', 3), ('vi', 4), ('vii', 1)]
# Create a defaultdict 'd' to store lists of roll numbers for each class
d = defaultdict(list)
# Loop through the tuples in 'class_roll'
for k, v in class_roll:
# Append the roll number 'v' to the list associated with class 'k' in 'd'
d[k].append(v)
# Sort the items (key-value pairs) in 'd' and print the result
print(sorted(d.items()))
Sample Output:
[('v', [1, 3]), ('vi', [2, 4]), ('vii', [1])]
Flowchart:
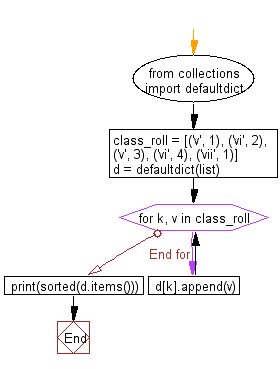
Python Code Editor:
Previous: Write a Python program to create an instance of an OrderedDict using a given dictionary. Sort the dictionary during the creation and print the members of the dictionary in reverse order.
Next: Write a Python program to compare two unordered lists (not sets).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics