Python: Create a deque and append few elements to the left and right, then remove some elements from the left, right sides and reverse the deque
Write a Python program to create a deque and append a few elements to the left and right. Next, remove some elements from the left and right sides and reverse the deque.
Sample Solution:
Python Code:
# Import the collections module to use the deque data structure
import collections
# Create a deque named 'deque_colors' with initial elements "Red", "Green", and "White"
deque_colors = collections.deque(["Red", "Green", "White"])
# Print the original deque
print(deque_colors)
# Append "Pink" to the left of the deque
print("\nAdding to the left: ")
deque_colors.appendleft("Pink")
print(deque_colors)
# Append "Orange" to the right of the deque
print("\nAdding to the right: ")
deque_colors.append("Orange")
print(deque_colors)
# Remove and print the element from the right of the deque
print("\nRemoving from the right: ")
deque_colors.pop()
print(deque_colors)
# Remove and print the element from the left of the deque
print("\nRemoving from the left: ")
deque_colors.popleft()
print(deque_colors)
# Reverse the order of elements in the deque
print("\nReversing the deque: ")
deque_colors.reverse()
print(deque_colors)
Sample Output:
deque(['Red', 'Green', 'White']) Adding to the left: deque(['Pink', 'Red', 'Green', 'White']) Adding to the right: deque(['Pink', 'Red', 'Green', 'White', 'Orange']) Removing from the right: deque(['Pink', 'Red', 'Green', 'White']) Removing from the left: deque(['Red', 'Green', 'White']) Reversing the deque: deque(['White', 'Green', 'Red'])
Flowchart:
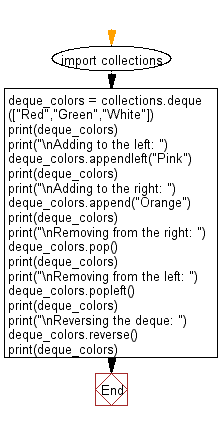
Python Code Editor:
Previous: Write a Python program that accepts number of subjects, subject names and marks. Input number of subjects and then subject name, marks separated by a space in next line. Print subject name and marks in order of its first occurrence.
Next: Write a Python program to create a deque from an existing iterable object.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics