Python Cyber Security – Generate random passwords of specified length
2. Random Password Generator
Write a Python program that defines a function to generate random passwords of a specified length. The function takes an optional parameter length, which is set to 8 by default. If no length is specified by the user, the password will have 8 characters.
Sample Solution:
Python Code:
import random
import string
def generate_password(length=8):
# Define the characters to use in the password
all_characters = string.ascii_letters + string.digits + string.punctuation
# Use the random module to generate the password
password = ''.join(random.choice(all_characters) for i in range(length))
return password
password_length_str = input("Input the desired length of your password:")
if password_length_str:
password_length = int(password_length_str)
else:
password_length = 8
password = generate_password(password_length)
print(f"Generated password is: {password}")
Sample Output:
Input the desired length of your password: Generated password is: &bMFdgNo Input the desired length of your password: 4 Generated password is: i$3H Input the desired length of your password: 7 Generated password is: (`9z}Q% Input the desired length of your password: 15 Generated password is: ?A5]3&h1e-:9OkR
Flowchart:
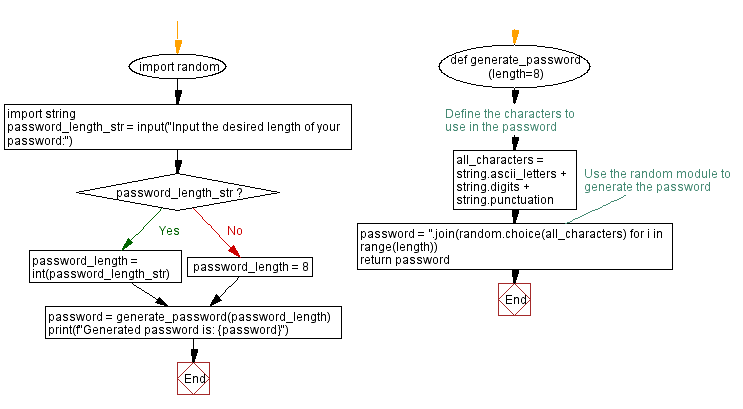
For more Practice: Solve these Related Problems:
- Write a Python program that generates a random password of a specified length (default 8) using a combination of uppercase, lowercase, digits, and punctuation.
- Write a Python function that accepts an optional length parameter and returns a random password, ensuring that at least one character from each category is included.
- Write a Python script to generate 5 random passwords of variable lengths and print them in a formatted table.
- Write a Python program to allow the user to specify the character set for the password and then generate a random password of the given length.
Go to:
Previous: Hash Password String using SHA-256 Algorithm.
Next: Check password strength.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.