Python Linked List: Delete the first item from a singly linked list
6. Delete First Item in Singly Linked List
Write a Python program to delete the first item from a singly linked list.
Sample Solution:
Python Code:
class Node:
# Singly linked node
def __init__(self, data=None):
self.data = data
self.next = None
class singly_linked_list:
def __init__(self):
# Createe an empty list
self.tail = None
self.head = None
self.count = 0
def append_item(self, data):
#Append items on the list
node = Node(data)
if self.head:
self.head.next = node
self.head = node
else:
self.tail = node
self.head = node
self.count += 1
def delete_item(self, data):
# Delete an item from the list
current = self.tail
prev = self.tail
while current:
if current.data == data:
if current == self.tail:
self.tail = current.next
else:
prev.next = current.next
self.count -= 1
return
prev = current
current = current.next
def iterate_item(self):
# Iterate the list.
current_item = self.tail
while current_item:
val = current_item.data
current_item = current_item.next
yield val
items = singly_linked_list()
items.append_item('PHP')
items.append_item('Python')
items.append_item('C#')
items.append_item('C++')
items.append_item('Java')
print("Original list:")
for val in items.iterate_item():
print(val)
print("\nAfter removing the first item from the list:")
items.delete_item('PHP')
for val in items.iterate_item():
print(val)
Sample Output:
Original list: PHP Python C# C++ Java After removing the first item from the list: Python C# C++ Java
Flowchart:
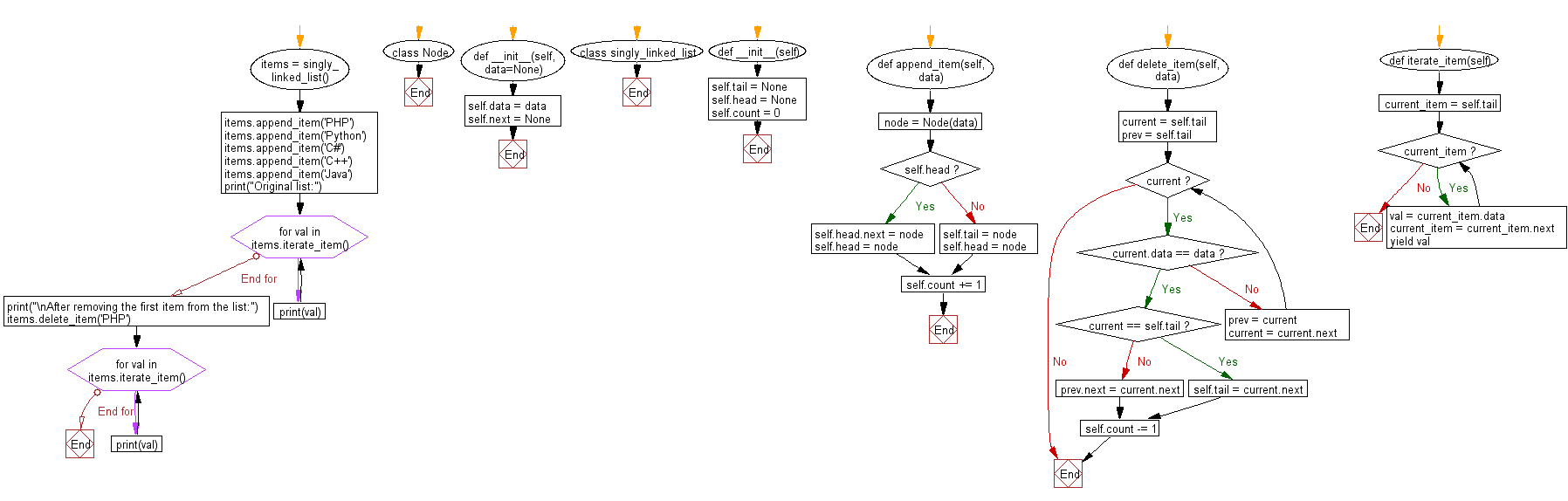
For more Practice: Solve these Related Problems:
- Write a Python program to delete the first node of a singly linked list and then iterate over the modified list to print the remaining values.
- Write a Python script to remove the head node from a singly linked list and return the new head, ensuring proper pointer updates.
- Write a Python program to implement deletion of the first element and verify that the list becomes empty if it contained only one node.
- Write a Python function to delete the first node in a singly linked list and update a size counter accordingly.
Go to:
Previous: Write a Python program to set a new value of an item in a singly linked list using index value.
Next: Write a Python program to delete the last item from a singly linked list.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.