Python Data Structures and Algorithms - Recursion: Calculate the value of 'a' to the power 'b'
Python Recursion: Exercise-10 with Solution
Write a Python program to calculate the value of 'a' to the power of 'b' using recursion.
Test Data:
(power(3,4) -> 81
Sample Solution:
Python Code:
# Define a function named power that calculates the result of 'a' raised to the power of 'b'
def power(a, b):
# Check if 'b' is 0 (base case for power function)
if b == 0:
# If 'b' is 0, return 1 (any number raised to the power of 0 is 1)
return 1
# Check if 'a' is 0 (base case for power function)
elif a == 0:
# If 'a' is 0, return 0 (0 raised to any power is 0)
return 0
# Check if 'b' is 1 (base case for power function)
elif b == 1:
# If 'b' is 1, return 'a' (any number raised to the power of 1 is the number itself)
return a
else:
# If none of the base cases is met, recursively call the power function
# to calculate 'a' multiplied by the result of 'a' raised to the power of 'b-1'
return a * power(a, b - 1)
# Print the result of calling the power function with the input values 3 and 4
print(power(3, 4))
Sample Output:
81
Flowchart:
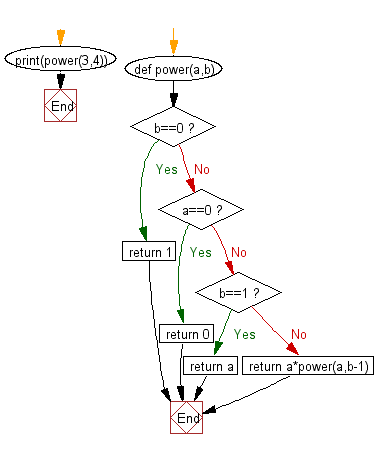
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to calculate the geometric sum of n-1.
Next: Write a Python program to find the greatest common divisor (gcd) of two integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/data-structures-and-algorithms/python-recursion-exercise-10.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics