Python Data Structures and Algorithms - Recursion: Factorial of a non-negative integer
Python Recursion: Exercise-4 with Solution
Write a Python program to get the factorial of a non-negative integer using recursion.
Sample Solution:
Python Code:
# Define a function named factorial that calculates the factorial of a given number 'n'
def factorial(n):
# Check if 'n' is less than or equal to 1
if n <= 1:
# If 'n' is 1 or less, return 1 (base case for factorial)
return 1
else:
# If 'n' is greater than 1, recursively call the factorial function
# to calculate the factorial of (n - 1) and multiply the result by 'n'
return n * factorial(n - 1)
# Print the result of calling the factorial function with the input value 5
print(factorial(5))
Sample Output:
120
Flowchart:
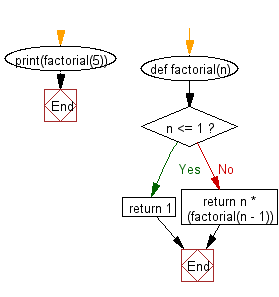
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program of recursion list sum.
Next: Write a Python program to solve the Fibonacci sequence using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/data-structures-and-algorithms/python-recursion-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics