Python Data Structures and Algorithms - Recursion: Sum of n+(n-2)+(n-4)...
7. Sum of Series n + (n-2) + (n-4) ... Using Recursion
Write a Python program to calculate the sum of the positive integers of n+(n-2)+(n-4)... (until n-x =< 0) using recursion.
Sample Solution:
Python Code:
# Define a function named sum_series that calculates the sum of a series of numbers
def sum_series(n):
# Check if 'n' is less than 1 (base case for the series)
if n < 1:
# If 'n' is less than 1, return 0 (base case value for the sum)
return 0
else:
# If 'n' is greater than or equal to 1, calculate the sum of 'n' and
# recursively call the sum_series function with 'n - 2'
return n + sum_series(n - 2)
# Print the result of calling the sum_series function with the input value 6
print(sum_series(6))
# Print the result of calling the sum_series function with the input value 10
print(sum_series(10))
Sample Output:
12 30
Flowchart:
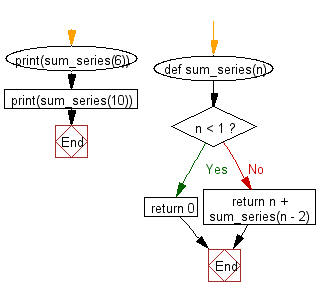
For more Practice: Solve these Related Problems:
- Write a Python program to recursively compute the sum of the series n, n-2, n-4, ... until the term is less than or equal to 0.
- Write a Python program to implement a recursive function that sums the series of positive integers decrementing by 2 at each step.
- Write a Python program to calculate the sum of the series n + (n-2) + ... using recursion and verify it with an iterative approach.
- Write a Python program to use recursion to sum a decreasing sequence starting from n with a step of 2, stopping when the term is non-positive.
Go to:
Previous: Write a Python program to get the sum of a non-negative integer.
Next: Write a Python program to calculate the harmonic sum of n-1.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.