Python Data Structures and Algorithms - Recursion: Calculate the geometric sum
9. Geometric Series Sum Using Recursion
Write a Python program to calculate the geometric sum up to 'n' terms.
Note : In mathematics, a geometric series is a series with a constant ratio between successive terms.
Sample Solution:
Python Code:
# Define a function named geometric_sum that calculates the geometric sum up to 'n' terms
def geometric_sum(n):
# Check if 'n' equals 0, which is the base case for the geometric sum
if n == 0: # Corrected base case condition
# If 'n' equals 0, return 1 as the geometric sum in this case is 1
return 1
else:
# If 'n' is not 0, calculate the term in the geometric series (1 / 2^n) and add it to
# the result of recursively calling the geometric_sum function with 'n - 1'
return 1 / (pow(2, n)) + geometric_sum(n - 1)
# Print the result of calling the geometric_sum function with the input value 7
print(geometric_sum(7))
# Print the result of calling the geometric_sum function with the input value 4
print(geometric_sum(4))
Sample Output:
1.9921875 1.9375
Flowchart:
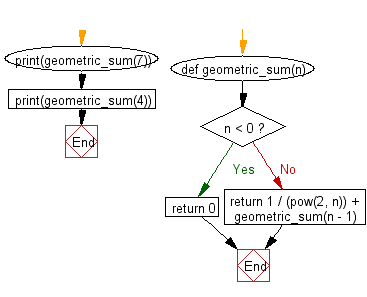
For more Practice: Solve these Related Problems:
- Write a Python program to recursively compute the sum of a geometric series given the first term and common ratio for n terms.
- Write a Python program to implement a recursive function that calculates the sum of a geometric progression with a constant ratio.
- Write a Python program to compute the geometric sum using recursion and verify the result with the geometric series formula.
- Write a Python program to implement recursion for the sum of a geometric series and handle cases where the common ratio is less than one.
Go to:
Previous: Write a Python program to calculate the harmonic sum of n-1.
Next: Write a Python program to calculate the value of 'a' to the power 'b'.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.