Python: Sequential search
2. Sequential Search
Write a Python program for sequential search.
Sequential Search: In computer science, linear search or sequential search is a method for finding a particular value in a list that checks each element in sequence until the desired element is found or the list is exhausted. The list need not be ordered.
Sample Solution:
Python Code:
def Sequential_Search(dlist, item):
pos = 0
found = False
while pos < len(dlist) and not found:
if dlist[pos] == item:
found = True
else:
pos = pos + 1
return found, pos
print(Sequential_Search([11,23,58,31,56,77,43,12,65,19],31))
Sample Output:
(True, 3)
Flowchart:
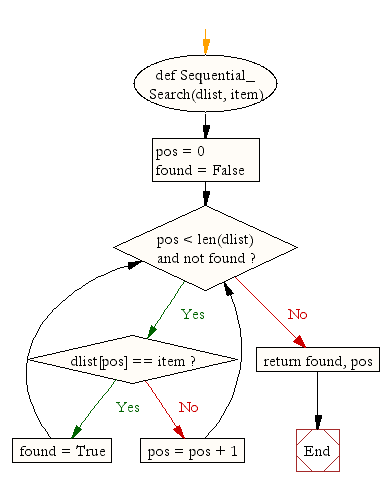
For more Practice: Solve these Related Problems:
- Write a Python program to implement a sequential search that returns a tuple containing a boolean result and the index of the found element.
- Write a Python program to perform linear search on a list of mixed data types and return the position of the target if it exists.
- Write a Python function to implement sequential search that also counts and prints the number of iterations taken.
- Write a Python program to modify the sequential search so that it stops after checking half the list if the element is not found.
Go to:
Previous: Write a Python program for binary search.
Next: Write a Python program for binary search for an ordered list.
Python Code Editor :
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.