Python: Sort an unsorted array numbers using Wiggle sort
24. Wiggle Sort
Write a Python program to sort an unsorted array of numbers using Wiggle sort.
Wiggle Sort:
Given an unsorted array nums, reorder it in-place such that nums[0] <= nums[1] >= nums[2] <= nums[3]....
For example, given nums = [3, 5, 2, 1, 6, 4], one possible answer is [1, 6, 2, 5, 3, 4].
Sample Solution:
Python Code:
def wiggle_sort(arra_nums):
for i, _ in enumerate(arra_nums):
if (i % 2 == 1) == (arra_nums[i - 1] > arra_nums[i]):
arra_nums[i - 1], arra_nums[i] = arra_nums[i], arra_nums[i - 1]
return arra_nums
print("Input the array elements: ")
arra_nums = list(map(int, input().split()))
print("Original unsorted array:")
print(arra_nums)
print("The said array after applying Wiggle sort:")
print(wiggle_sort(arra_nums))
Sample Output:
Input the array elements: 1 5 2 3 4 6 Original unsorted array: [1, 5, 2, 3, 4, 6] The said array after applying Wiggle sort: [1, 5, 2, 4, 3, 6]
Flowchart:
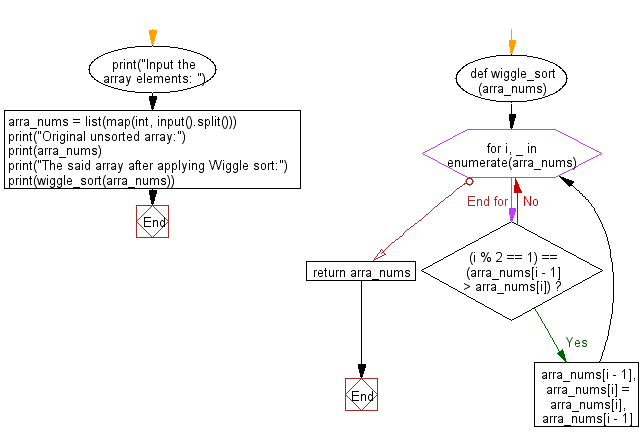
For more Practice: Solve these Related Problems:
- Write a Python program to implement wiggle sort on an unsorted array and then verify the pattern nums[0] ≤ nums[1] ≥ nums[2] ≤ ... holds.
- Write a Python script to rearrange a list into wiggle order and then output the transformed list along with validation of the pattern.
- Write a Python program to use wiggle sort on a list of integers and then count the number of positions where the condition is satisfied.
- Write a Python function to implement an in-place wiggle sort and then print the list before and after sorting.
Go to:
Previous: Write a Python program to sort a list of elements using Tree sort.
Next: Write a Python program to sort unsorted numbers using Timsort.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.