Python: Insertion sort
Python Search and Sorting : Exercise-6 with Solution
Write a Python program to sort a list of elements using the insertion sort algorithm.
Note: According to Wikipedia "Insertion sort is a simple sorting algorithm that builds the final sorted array (or list) one item at a time. It is much less efficient on large lists than more advanced algorithms such as quicksort, heapsort, or merge sort."
Pictorial Presentation: Insertion Sort
Sample Solution:
Python Code:
def insertionSort(nlist):
for index in range(1,len(nlist)):
currentvalue = nlist[index]
position = index
while position>0 and nlist[position-1]>currentvalue:
nlist[position]=nlist[position-1]
position = position-1
nlist[position]=currentvalue
nlist = [14,46,43,27,57,41,45,21,70]
insertionSort(nlist)
print(nlist)
Sample Output:
[14, 21, 27, 41, 43, 45, 46, 57, 70]
Flowchart :
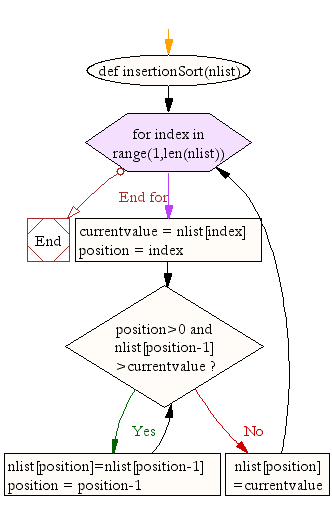
Python Code Editor :
Contribute your code and comments through Disqus.
Previous: Write a Python program to sort a list of elements using the selection sort algorithm.
Next: Write a Python program to sort a list of elements using shell sort algorithm.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/data-structures-and-algorithms/python-search-and-sorting-exercise-6.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics