Python: Add 5 seconds with the current time
10. Add 5 Seconds
Write a Python program to add 5 seconds to the current time.
Sample Solution:
Python Code:
# Import the datetime module
import datetime
# Get the current date and time and assign it to the variable 'x' using datetime.datetime.now()
x = datetime.datetime.now()
# Add a timedelta of 5 seconds to the current date and time 'x' and assign the result to the variable 'y'
y = x + datetime.timedelta(0,5)
# Print the time component of the current date and time 'x'
print(x.time())
# Print the time component of the date and time 'y' (which is 'x' + 5 seconds)
print(y.time())
Output:
12:37:43.350241 12:37:48.350241
Explanation:
In the exercise above,
- The code imports the "datetime" module, which provides functionalities to work with dates and times.
- Get the current date and time:
- It retrieves the current date and time using "datetime.datetime.now()" and assigns it to the variable 'x'.
- Adding Time Delta:
- It creates a time delta of 5 seconds using "datetime.timedelta(0,5)" and adds it to the current date and time 'x'. The result is stored in the variable 'y'.
- Finally it prints the time component of the current date and time 'x' using "x.time()".
- Next it prints the time component of the date and time 'y' (which is 'x' + 5 seconds) using "y.time()".
Flowchart:
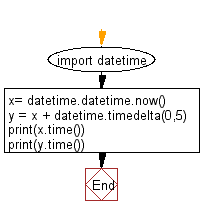
For more Practice: Solve these Related Problems:
- Write a Python program to add 5 seconds to the current time and then print both the original and updated times in "HH:MM:SS" format.
- Write a Python script to increment the current time by 5 seconds repeatedly for 10 iterations and display each new time.
- Write a Python program that adds 5 seconds to a given time string and then converts the result back to a time object.
- Write a Python function to add 5 seconds to the current time, then compute the difference in seconds between the new time and a fixed reference time.
Go to:
Previous: Write a Python program to print next 5 days starting from today.
Next: Write a Python program to convert Year/Month/Day to Day of Year.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.