Python: Get week number
Python Datetime: Exercise-13 with Solution
Write a Python program to get the week number.
Sample Solution:
Python Code:
# Import the datetime module
import datetime
# Create a datetime object representing the date 'June 16, 2015' and retrieve its ISO calendar week number
# The isocalendar() method returns a tuple containing year, week number, and weekday
# We access the second element of the tuple, which represents the week number, using [1]
print(datetime.date(2015, 6, 16).isocalendar()[1])
Output:
25
Explanation:
In the exercise above,
- The code imports the datetime module.
- Get ISO Calendar week number:
- It creates a "datetime" object representing the date 'June 16, 2015' using datetime.date(2015, 6, 16).
- Then, it retrieves the ISO calendar week number of that date using the "isocalendar()" method.
- The "isocalendar()" method returns a tuple containing year, week number, and weekday.
- By accessing the second element of the tuple ([1]), which represents the week number, it obtains the ISO calendar week number.
- Printing Results:
- It prints the ISO calendar week number of the date 'June 16, 2015' using "print()".
Flowchart:
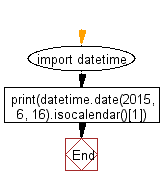
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to get current time in milliseconds.
Next: Write a Python program to find the date of the first Monday of a given week.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/date-time-exercise/python-date-time-exercise-13.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics