Python: Find the date of the first Monday of a given week
Python Datetime: Exercise-14 with Solution
Write a Python program to find the date of the first Monday of a given week.
Sample Solution:
Python Code:
# Import the time module
import time
# Convert the given date string '2015 50 1' into a struct_time object using time.strptime()
# The date string format is specified as '%Y %W %w', representing year, ISO week number, and weekday respectively
# Then, convert the struct_time object to a string representation of the date and time using time.asctime()
# and print it
print(time.asctime(time.strptime('2015 50 1', '%Y %W %w')))
Output:
Mon Dec 14 00:00:00 2015
Explanation:
In the exercise above,
- The code imports the "time" module.
- Parsing the Date String:
- It takes the date string '2015 50 1' representing the year, ISO week number, and weekday respectively.
- The "strptime()" function from the "time" module parses this date string into a struct_time object according to the specified format '%Y %W %w', where:
- %Y represents the year with the century as a decimal number.
- %W represents the ISO week number of the year.
- %w represents the weekday as a decimal number, where Monday is 0 and Sunday is 6.
- Formatting and printing results:
- The parsed "struct_time" object is then converted back to a string representation of the date and time using the "asctime()" function from the "time" module.
- Finally, it prints the string representation of the parsed date and time.
Flowchart:
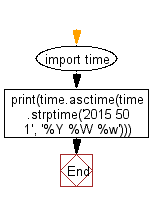
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to get week number.
Next: Write a Python program to select all the Sundays of a specified year.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/date-time-exercise/python-date-time-exercise-14.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics