Python: Get the date of the last Tuesday
Python Datetime: Exercise-19 with Solution
Write a Python program to get the date of the last Tuesday.
Sample Solution:
Python Code:
# Import the date class from the datetime module
from datetime import date
# Import the timedelta class from the datetime module
from datetime import timedelta
# Get the current date and assign it to the variable 'today'
today = date.today()
# Calculate the offset needed to go back to the most recent Tuesday
# This is done by finding the difference between the current weekday and Tuesday (1), and taking modulo 7
offset = (today.weekday() - 1) % 7
# Calculate the date of the most recent Tuesday by subtracting the offset from the current date
last_tuesday = today - timedelta(days=offset)
# Print the date of the most recent Tuesday
print(last_tuesday)
Output:
2017-05-02
Explanation:
In the exercise above,
- The code imports the "date" class and the "timedelta" class from the "datetime" module.
- Get current date:
- It retrieves the current date using the "date.today()" method and assigns it to the variable 'today'.
- Calculating Offset for most recent Tuesday:
- It calculates the offset needed to go back to the most recent Tuesday from the current date.
- This is done by finding the difference between the current weekday (today.weekday()) and Tuesday (1, where Monday is 0), then taking modulo 7 to ensure the offset loops back to Tuesday if the current day is Tuesday.
- Find the most recent Tuesday:
- It calculates the date of the most recent Tuesday by subtracting the calculated offset from the current date using "timedelta(days=offset)".
- Finally it prints the date of the most recent Tuesday.
Flowchart:
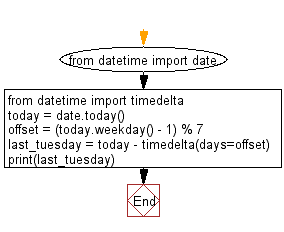
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to get days between two dates.
Next: Write a Python program to test the third Tuesday of a month.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/date-time-exercise/python-date-time-exercise-19.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics