Python: Get the last day of a specified year and month
Write a Python program to get the last day of a specified year and month.
Sample Solution:
Python Code:
# Import the calendar module
import calendar
# Define the year and month for which we want to find the number of days
year = 2015
month = 2
# Use the monthrange() function from the calendar module to get the number of days in the specified month
# The [1] index of the returned tuple contains the number of days in the month
print(calendar.monthrange(year, month)[1])
Output:
28
Explanation:
In the exercise above,
- The code imports the "calendar" module.
- Define Year and Month:
- It defines variables 'year' and 'month' with the values 2015 and 2, respectively. These represent the year and month for which we want to find the number of days.
- Calculating the number of days in the month:
- It uses the "monthrange()" function from the "calendar" module to get information about the specified month (month) in the specified year (year).
- The "monthrange()" function returns a tuple containing two values:
- The first value is the weekday of the first day of the month (0 for Monday, 1 for Tuesday, etc.).
- The second value is the number of days in the month.
- By accessing the second value of the tuple ([1]), the code retrieves the number of days in the specified month.
- Finally it prints the number of days in the specified month using "print()".
Flowchart:
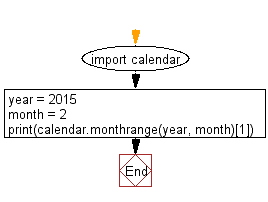
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to test the third Tuesday of a month.
Next: Write a Python program to get the number of days of a given month and year.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics