Python: Get the number of days of a given month and year
Write a Python program to get the number of days in a given month and year.
Sample Solution:
Python Code:
# Import the monthrange function from the calendar module
from calendar import monthrange
# Define the year and month for which we want to find information
year = 2016
month = 2
# Use the monthrange function to get information about the specified month and year
# The function returns a tuple containing the weekday of the first day of the month (0 for Monday, 1 for Tuesday, etc.)
# and the number of days in the month.
print(monthrange(year, month))
Output:
(0, 29)
Explanation:
In the exercise above,
- The code imports the "monthrange()" function from the "calendar" module.
- Define Year and Month:
- It defines variables 'year' and 'month' with the values 2016 and 2, respectively.
- Month information:
- It uses the "monthrange()" function to get information about the specified month (month) in the specified year (year).
- The "monthrange()" function returns a tuple containing two values:
- The first value is the weekday of the first day of the month (0 for Monday, 1 for Tuesday, etc.).
- The second value is the number of days in the month.
- It prints the tuple returned by the "monthrange()" function, which contains the weekday of the first day of the month and the number of days in the month.
Flowchart:
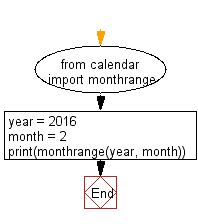
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to get the last day of a specified year and month.
Next: Write a Python program to add a month with a specified date.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics