Python: Count the number of Monday of the 1st day of the month between two years
Python Datetime: Exercise-24 with Solution
Write a Python program to count the number of Mondays on the 1st day of the month from 2015 to 2016.
Sample Solution:
Python Code:
# Import the datetime module
import datetime
# Import the datetime class from the datetime module
from datetime import datetime
# Initialize a counter to count occurrences of Monday being the first day of the month
monday1 = 0
# Define a range of months from 1 to 12
months = range(1, 13)
# Iterate over years from 2015 to 2016 (inclusive)
for year in range(2015, 2017):
# Iterate over each month in the range
for month in months:
# Check if the first day of the month (year, month, 1) falls on a Monday (weekday() == 0)
if datetime(year, month, 1).weekday() == 0:
# If the first day of the month is a Monday, increment the counter
monday1 += 1
# Print the total count of occurrences where Monday is the first day of the month
print(monday1)
Output:
3
Explanation:
In the exercise above,
- The code imports the "datetime" module, which provides functionalities for dates and times. It also imports the "datetime" class from the "datetime" module.
- Initializing variables:
- It initializes a variable 'monday1' to count occurrences where Monday is the first day of the month. This is initially set to 0.
- Iterating over years and months:
- It iterates over the years from 2015 to 2016 (inclusive) using a for loop.
- Within each year, it iterates over each month (from 1 to 12) using another for loop.
- Check first day of each month:
- For each combination of year and month, it checks if the first day of that month falls on a Monday (weekday() == 0) using the "weekday()" method of the "datetime" object.
- If the first day of the month is a Monday, it increments the 'monday1' counter.
- Finally it prints the total count of occurrences where Monday is the first day of the month.
Flowchart:
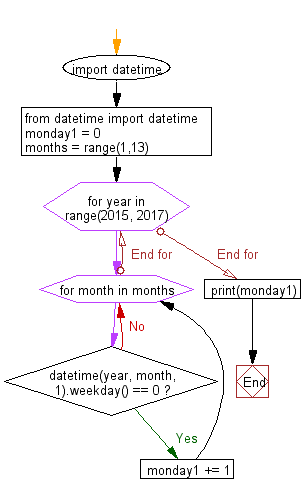
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to add a month with a specified date.
Next: Write a Python program to print a string five times, delay three seconds.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/date-time-exercise/python-date-time-exercise-24.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics