Python: Create 12 fixed dates from a specified date over a given period
Python Datetime: Exercise-27 with Solution
Write a Python program to create 12 fixed dates from a specified date over a given period. The difference between two dates is 20.
Sample Solution:
Python Code:
# Import the datetime module
import datetime
# Define a function named every_20_days that takes a date object (date) as input
def every_20_days(date):
# Print the starting date
print('Starting Date: {d}'.format(d=date))
# Print a message indicating the next 12 days
print("Next 12 days :")
# Execute a loop that repeats 12 times
for _ in range(12):
# Add 20 days to the current date
date = date + datetime.timedelta(days=20)
# Print the resulting date
print('{d}'.format(d=date))
# Define a date object representing August 1, 2016
dt = datetime.date(2016, 8, 1)
# Call the every_20_days function with the specified date object
every_20_days(dt)
Output:
Starting Date: 2016-08-01 Next 12 days : 2016-08-21 2016-09-10 2016-09-30 2016-10-20 2016-11-09 2016-11-29 2016-12-19 2017-01-08 2017-01-28 2017-02-17 2017-03-09 2017-03-29
Explanation:
In the exercise above,
- The code imports the "datetime" module.
- Define the function:
- It defines a function named "every_20_days()" that takes a date object (date) as input.
- Within this function,
- It prints the starting date passed to the function.
- It prints a message indicating the next 12 days.
- It executes a loop that repeats 12 times.
- Inside the loop, it adds 20 days to the current date (date) using the "timedelta()" function from the "datetime" module.
- It prints the resulting date.
- Initializing the date object:
- It initializes a date object 'dt' representing August 1, 2016.
- Call the function:
- It calls the "every_20_days()" function with the specified date object 'dt' as an argument.
Flowchart:
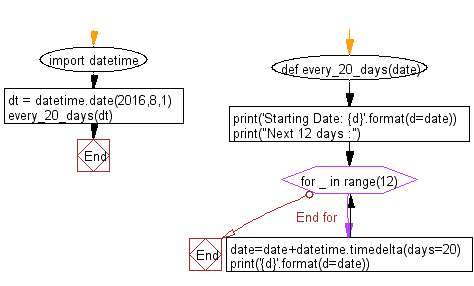
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program calculates the date six months from the current date using the datetime module.
Next: Write a Python program to get the dates 30 days before and after from the current date.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/date-time-exercise/python-date-time-exercise-27.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics