Python: Get the dates 30 days before and after from the current date
28. 30 Days Before/After
Write a Python program to get the dates 30 days before and after today.
Sample Solution:-
Python Code:
# Import the date and timedelta classes from the datetime module
from datetime import date, timedelta
# Get the current date and convert it to ISO 8601 format
current_date = date.today().isoformat()
# Calculate the date 30 days before the current date and convert it to ISO 8601 format
days_before = (date.today() - timedelta(days=30)).isoformat()
# Calculate the date 30 days after the current date and convert it to ISO 8601 format
days_after = (date.today() + timedelta(days=30)).isoformat()
# Print the current date, 30 days before the current date, and 30 days after the current date
print("\nCurrent Date: ", current_date)
print("30 days before current date: ", days_before)
print("30 days after current date : ", days_after)
Output:
Current Date: 2017-05-06 30 days before current date: 2017-04-06 30 days after current date : 2017-06-05
Explanation:
In the exercise above,
- The code imports the "date" and "timedelta" classes from the "datetime" module.
- Calculating dates:
- It calculates three dates:
- current_date: It retrieves the current date using the "date.today()" method, converts it to the ISO 8601 format using the "isoformat()" method, and assigns it to the variable 'current_date'.
- days_before: It calculates the date 30 days before the current date using the "timedelta(days=30)" function to subtract 30 days from the current date, converts it to the ISO 8601 format using the "isoformat()" method, and assigns it to the variable 'days_before'.
- days_after: It calculates the date 30 days after the current date using the "timedelta(days=30)" function to add 30 days to the current date, converts it to the ISO 8601 format using the "isoformat()" method, and assigns it to the variable 'days_after'.
- Finally it prints the current date, the date 30 days before the current date, and the date 30 days after the current date using the "print()" function.
Flowchart:
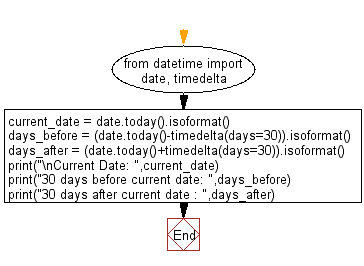
For more Practice: Solve these Related Problems:
- Write a Python program to calculate the date exactly 30 days before today and 30 days after today, then display both dates.
- Write a Python script to output a tuple containing the date 30 days prior and 30 days later than the current date.
- Write a Python function to generate and print the dates 30 days before and after a given date in "YYYY-MM-DD" format.
- Write a Python program to compute and compare the weekday names for the dates 30 days before and 30 days after today.
Go to:
Previous: Write a Python program to create 12 fixed dates from a specified date over a given period. The difference between two dates will be 20.
Next: Write a Python program to get the GMT and local current time.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.