Python: Convert a string to datetime
3. String to Datetime Converter
Write a Python program to convert a string to datetime.
Sample Solution:
Python Code:
# Import the datetime class from the datetime module
from datetime import datetime
# Parse the given date string 'Jul 1 2014 2:43PM' into a datetime object
# using the specified format '%b %d %Y %I:%M%p'
date_object = datetime.strptime('Jul 1 2014 2:43PM', '%b %d %Y %I:%M%p')
# Print the parsed datetime object
print(date_object)
Output:
2014-07-01 14:43:00
Explanation:
In the exercise above,
- The code imports the "datetime" class from the "datetime" module.
- Parsing the Date string:
- It then uses the "datetime.strptime()" method to parse the given date string 'Jul 1 2014 2:43PM' into a datetime object.
- The format of the input date string is specified as '%b %d %Y %I:%M%p', which indicates the month abbreviated (%b), day of the month (%d), year (%Y), hour in 12-hour format (%I), minute (%M), and AM/PM indicator (%p).
- Printing Results:
- Finally, it prints the parsed datetime object using the "print()" function.
Flowchart:
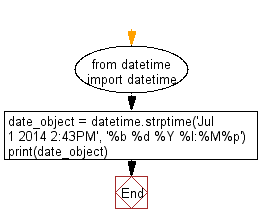
For more Practice: Solve these Related Problems:
- Write a Python program to convert a string in the format "DD-MM-YYYY HH:MM" to a datetime object and then display it in ISO format.
- Write a Python function that accepts a list of date strings in various formats and converts them all to datetime objects.
- Write a Python script to parse a date string with timezone information and convert it to a UTC datetime object.
- Write a Python program to convert a string like "March 15, 2022 08:30AM" to datetime and then extract the hour and minute.
Go to:
Previous: Write a Python program to determine whether a given year is a leap year.
Next: Write a Python program to get the current time.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.