Python: Generate a date and time as a string
Python Datetime: Exercise-41 with Solution
Write a Python program to generate a date and time as a string.
Sample Solution:
Python Code:
# Import the datetime module
import datetime
# Get the current date and time
now = datetime.datetime.now()
# Convert the current date and time into a formatted string
# Date and time in string format: 2016-11-05 11:24:24 PM
datestr = "# In string: " + now.strftime("%Y-%m-%d %H:%M:%S %p") + "\n"
# Print an empty line
print()
# Print the formatted string containing the current date and time
print(datestr)
# Print an empty line
print()
Output:
# In string: 2017-05-08 14:24:37 PM
Explanation:
In the exercise above,
- The code imports the "datetime" module.
- It gets the current date and time using "datetime.datetime.now()" function and assigns it to the variable 'now'.
- It converts the current date and time into a formatted string using the "strftime()" method, which formats a "datetime" object as a string according to the given format string. In this case, it formats the date and time as "YYYY-MM-DD HH:MM:SS AM/PM" format.
- It constructs a string 'datestr' containing the formatted date and time preceded by a note, and assigns it to 'datestr'.
- It prints the string 'datestr', which contains the formatted current date and time.
Flowchart:
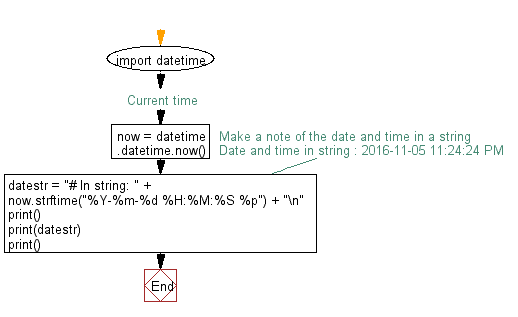
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to get the current date time information.
Next: Write a Python program to display formatted text output of a month and start weeks on Sunday.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/date-time-exercise/python-date-time-exercise-41.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics