Python: Display formatted text output of a month and start weeks on Sunday
42. Formatted Month Calendar
Write a Python program to display formatted text output of a month and start the week on Sunday.
Sample Solution:
Python Code:
# Import the calendar module
import calendar
# Create a TextCalendar object starting from Sunday as the first day of the week
cal = calendar.TextCalendar(calendar.SUNDAY)
# Print the header for the calendar for the first month of 2022
print('First Month - 2022')
# Print the calendar for January 2022
print(cal.prmonth(2022, 1))
Output:
First Month - 2022 January 2022 Su Mo Tu We Th Fr Sa 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 None
Explanation:
In the exercise above,
- The code imports the "calendar" module.
- It creates a "TextCalendar" object named 'cal' starting from Sunday as the first day of the week. This is specified by passing 'calendar.SUNDAY' as an argument.
- It prints a header indicating the month and year for the calendar being displayed.
- It prints the calendar for January 2022 using the "prmonth()" method of the "TextCalendar" object. This method generates a formatted calendar for a specific month and year.
Flowchart:
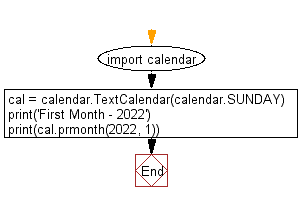
For more Practice: Solve these Related Problems:
- Write a Python program to display the calendar for a given month with the week starting on Sunday, formatted in a neat table.
- Write a Python script to print a month's calendar where each week is printed on a new line and the header shows the month and year.
- Write a Python function to output a formatted calendar for a specified month, highlighting the current day if it falls in that month.
- Write a Python program to generate a calendar for a month with custom spacing and alignment for each day, starting the week on Sunday.
Python Code Editor :
Contribute your code and comments through Disqus.
Previous: Write a python program to generate a date and time as a string.
Next: Write a Python program to print a 3-column calendar for an entire year.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.