Python: Print a 3-column calendar for an entire year
43. 3-Column Year Calendar
Write a Python program to print a 3-column calendar for an entire year.
Sample Solution:
Python Code:
# Import the calendar module
import calendar
# Create a TextCalendar object starting from Sunday as the first day of the week
cal = calendar.TextCalendar(calendar.SUNDAY)
# Specify the formatting parameters for the year calendar
# year: 2022
# column width: 2
# lines per week: 1
# number of spaces between month columns: 1
# 3: number of months per column
# Generate the formatted year calendar for 2022 using the specified parameters
print(cal.formatyear(2022, 2, 1, 1, 3))
Output:
2022 January February March Su Mo Tu We Th Fr Sa Su Mo Tu We Th Fr Sa Su Mo Tu We Th Fr Sa 1 1 2 3 4 5 1 2 3 4 5 2 3 4 5 6 7 8 6 7 8 9 10 11 12 6 7 8 9 10 11 12 9 10 11 12 13 14 15 13 14 15 16 17 18 19 13 14 15 16 17 18 19 16 17 18 19 20 21 22 20 21 22 23 24 25 26 20 21 22 23 24 25 26 23 24 25 26 27 28 29 27 28 27 28 29 30 31 30 31 April May June Su Mo Tu We Th Fr Sa Su Mo Tu We Th Fr Sa Su Mo Tu We Th Fr Sa 1 2 1 2 3 4 5 6 7 1 2 3 4 3 4 5 6 7 8 9 8 9 10 11 12 13 14 5 6 7 8 9 10 11 10 11 12 13 14 15 16 15 16 17 18 19 20 21 12 13 14 15 16 17 18 17 18 19 20 21 22 23 22 23 24 25 26 27 28 19 20 21 22 23 24 25 24 25 26 27 28 29 30 29 30 31 26 27 28 29 30 July August September Su Mo Tu We Th Fr Sa Su Mo Tu We Th Fr Sa Su Mo Tu We Th Fr Sa 1 2 1 2 3 4 5 6 1 2 3 3 4 5 6 7 8 9 7 8 9 10 11 12 13 4 5 6 7 8 9 10 10 11 12 13 14 15 16 14 15 16 17 18 19 20 11 12 13 14 15 16 17 17 18 19 20 21 22 23 21 22 23 24 25 26 27 18 19 20 21 22 23 24 24 25 26 27 28 29 30 28 29 30 31 25 26 27 28 29 30 31 October November December Su Mo Tu We Th Fr Sa Su Mo Tu We Th Fr Sa Su Mo Tu We Th Fr Sa 1 1 2 3 4 5 1 2 3 2 3 4 5 6 7 8 6 7 8 9 10 11 12 4 5 6 7 8 9 10 9 10 11 12 13 14 15 13 14 15 16 17 18 19 11 12 13 14 15 16 17 16 17 18 19 20 21 22 20 21 22 23 24 25 26 18 19 20 21 22 23 24 23 24 25 26 27 28 29 27 28 29 30 25 26 27 28 29 30 31 30 31
Explanation:
In the exercise above,
- The code imports the "calendar" module.
- It creates a "TextCalendar" object named 'cal' starting from Sunday as the first day of the week. This is specified by passing "calendar.SUNDAY" as an argument.
- It specifies the formatting parameters for generating the year calendar:
- Year: 2022
- Column width: 2 (Each day of the month will be displayed within a 2-character width)
- Lines per week: 1 (Each week will be displayed on a single line)
- Number of spaces between month columns: 1
- Number of months per column: 3 (Three months will be displayed side by side in each column)
- It generates and prints the formatted year calendar for 2022 using the "formatyear()" method of the "TextCalendar" object with the specified parameters.
Flowchart:
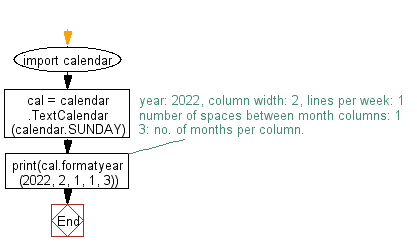
For more Practice: Solve these Related Problems:
- Write a Python program to print an entire year's calendar in a 3-column layout using the calendar module.
- Write a Python script to display a year’s calendar in three columns, ensuring that each month's header is properly centered.
- Write a Python function to generate a 3-column calendar for a given year and then print each month's calendar with borders.
- Write a Python program to create a multi-column calendar layout for an entire year and then export it as plain text.
Go to:
Previous: Write a Python program to display formatted text output of a month and start weeks on Sunday.
Next: Write a Python program to display a calendar for a locale.
Python Code Editor :
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.