Python: Display a calendar for a locale
Write a Python program to display a calendar for a locale.
Supported locale (locale –a):
students@ubuntu-1gb-nyc2-01:~$ locale -a
C , C.UTF-8, en_AG, en_AG.utf8, en_AU.utf8, en_BW.utf8, en_CA.utf8, en_DK.utf8,
en_GB.utf8, en_HK.utf8, en_IE.utf8, en_IN, en_IN.utf8, en_NG, en_NG.utf8, en_NZ.utf8
Sample Solution:
Python Code:
# Import the calendar module
import calendar
# Create a LocaleTextCalendar object with the locale set to 'en_AU.utf8' (Australian English)
cal = calendar.LocaleTextCalendar(locale='en_AU.utf8')
# Print the calendar for September 2025 in Australian English locale
print(cal.prmonth(2025, 9))
Output:
September 2025 Mo Tu We Th Fr Sa Su 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 None
Explanation:
In the exercise above,
- The code imports the "calendar" module.
- It creates a "LocaleTextCalendar object" named 'cal' with the locale set to 'en_AU.utf8', representing Australian English.
- It prints the calendar for September 2025 using the "prmonth()" method of the 'LocaleTextCalendar' object. This method generates a formatted calendar for a specific month and year. Since the locale is set to Australian English, the output will be in accordance with Australian English conventions.
Flowchart:
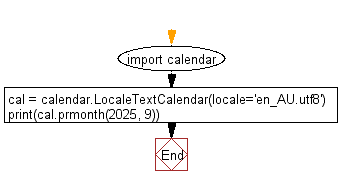
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to print a 3-column calendar for an entire year.
Next: Write a Python program to get the current week.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics