Python: Create a HTML calendar with data for a specific year and month
46. Calendar Creator
Write a Python program to create a HTML calendar with data for a specific year and month.
Sample Solution:
Python Code:
# Import the calendar module
import calendar
# Create an HTMLCalendar object with Monday as the first day of the week
htmlcal = calendar.HTMLCalendar(calendar.MONDAY)
# Generate and print the HTML-formatted calendar for December 2020
print(htmlcal.formatmonth(2020, 12))
HTML Code:
<table border="0" cellpadding="0" cellspacing="0" class="month">
<tr><th colspan="7" class="month">December 2020</th></tr>
<tr><th class="mon">Mon</th><th class="tue">Tue</th><th class="wed">Wed</th><th class="thu">Thu</th><th class=
"fri">Fri</th><th class="sat">Sat</th><th class="sun">Sun</th></tr>
<tr><td class="noday"> </td><td class="tue">1</td><td class="wed">2</td><td class="thu">3</td><td class="
fri">4</td><td class="sat">5</td><td class="sun">6</td></tr>
<tr><td class="mon">7</td><td class="tue">8</td><td class="wed">9</td><td class="thu">10</td><td class="fri">1
1</td><td class="sat">12</td><td class="sun">13</td></tr>
<tr><td class="mon">14</td><td class="tue">15</td><td class="wed">16</td><td class="thu">17</td><td class="fri
">18</td><td class="sat">19</td><td class="sun">20</td></tr>
<tr><td class="mon">21</td><td class="tue">22</td><td class="wed">23</td><td class="thu">24</td><td class="fri
">25</td><td class="sat">26</td><td class="sun">27</td></tr>
<tr><td class="mon">28</td><td class="tue">29</td><td class="wed">30</td><td class="thu">31</td><td class="nod
ay"> </td><td class="noday"> </td><td class="noday"> </td></tr>
</table>
Output:
December 2020 | ||||||
---|---|---|---|---|---|---|
Mon | Tue | Wed | Thu | Fri | Sat | Sun |
1 | 2 | 3 | 4 | 5 | 6 | |
7 | 8 | 9 | 10 | 1 1 | 12 | 13 |
14 | 15 | 16 | 17 | 18 | 19 | 20 |
21 | 22 | 23 | 24 | 25 | 26 | 27 |
28 | 29 | 30 | 31 |
Explanation:
In the exercise above,
- The code imports the "calendar" module, which provides functions for working with calendars.
- It creates an "HTMLCalendar" object named 'htmlcal' with Monday as the first day of the week, specified by passing "calendar.MONDAY" as an argument.
- It generates and prints the HTML-formatted calendar for December 2020 using the "formatmonth()" method of the 'HTMLCalendar' object. This method generates an HTML-formatted calendar for a specific month and year.
Flowchart:
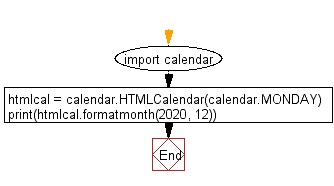
For more Practice: Solve these Related Problems:
- Write a Python program to generate an calendar for a specific month and year, embedding the calendar in a simple webpage.
- Write a Python script to create an file that displays a calendar for a given month with custom CSS styles.
- Write a Python function to output an calendar with clickable dates that link to a details page.
- Write a Python program to generate an interactive calendar for a specified month and then highlight today's date.
Go to:
Previous: Write a Python program to get the current week.
Next: Write a Python program display a list of the dates for the 2nd Saturday of every month for a given year.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.