Python: Display a list of the dates for the 2nd Saturday of every month for a given year
47. 2nd Saturday Dates
Write a Python program display a list of the dates for the 2nd Saturday of every month for a given year.
Sample Solution:
Python Code:
# Import the calendar module
import calendar
# Show every month
for month in range(1, 13):
# Generate the month calendar for the year 2020 and the current month
cal = calendar.monthcalendar(2020, month)
# Extract the first, second, and third weeks of the month
first_week = cal[0]
second_week = cal[1]
third_week = cal[2]
# If a Saturday is present in the first week, the second Saturday
# is in the second week. Otherwise, the second Saturday must be in the third week.
if first_week[calendar.SATURDAY]:
holi_day = second_week[calendar.SATURDAY]
else:
holi_day = third_week[calendar.SATURDAY]
# Print the month abbreviation and the date of the second Saturday of the month
print('%3s: %2s' % (calendar.month_abbr[month], holi_day))
Output:
Jan: 11 Feb: 8 Mar: 14 Apr: 11 May: 9 Jun: 13 Jul: 11 Aug: 8 Sep: 12 Oct: 10 Nov: 14 Dec: 12
Explanation:
In the exercise above,
- The code imports the "calendar" module.
- It iterates over each month (from January to December) using a "for" loop with the "range()" function.
- Inside the loop:
- It generates the month calendar for the year 2020 and the current month using the "monthcalendar()" function of the "calendar" module.
- It extracts the first, second, and third weeks of the month from the generated calendar.
- It checks if a Saturday is present in the first week. If so, it assigns the second Saturday of the month to the variable 'holi_day' from the second week. Otherwise, it assigns the second Saturday from the third week.
- It prints the month abbreviation and the date of the second Saturday of the month using string formatting with %3s for the month abbreviation and %2s for the date.
Flowchart:
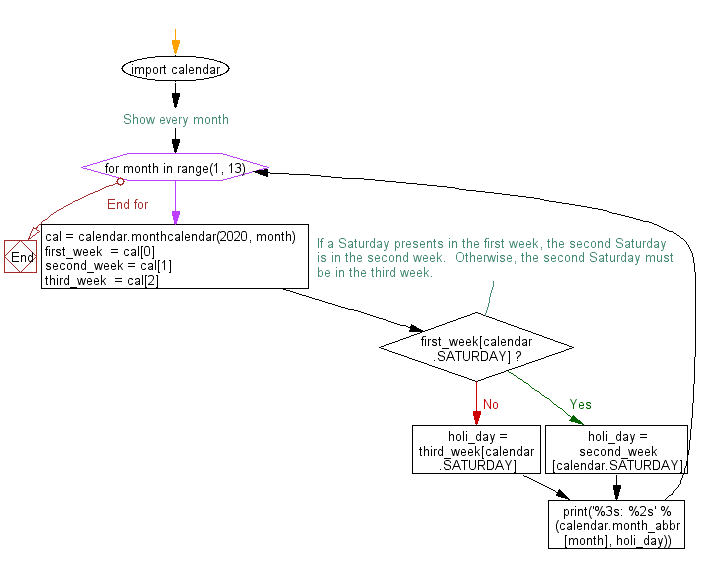
For more Practice: Solve these Related Problems:
- Write a Python program to list the dates for the second Saturday of every month for a given year.
- Write a Python script to compute and print the date of the 2nd Saturday for each month, then count how many fall on the 14th.
- Write a Python function to generate a list of dates corresponding to the second Saturday of each month and then verify them using the calendar module.
- Write a Python program to find and print all 2nd Saturday dates in a year and then check which of those are holidays using a provided list.
Go to:
Previous: Write a Python program to create a HTML calendar with data for a specific year and month.
Next: Write a Python program to display a simple, formatted calendar of a given year and month.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.