Python: Subtract five days from current date
5. Subtract Five Days
Write a Python program to subtract five days from the current date.
Sample Solution:
Python Code:
# Import the date class and the timedelta class from the datetime module
from datetime import date, timedelta
# Calculate the date 5 days before today's date
dt = date.today() - timedelta(5)
# Print the current date using date.today()
print('Current Date :', date.today())
# Print the date calculated 5 days before the current date
print('5 days before Current Date :', dt)
Output:
Current Date : 2017-05-05 5 days before Current Date : 2017-04-30
Explanation:
In the exercise above,
- The code imports the "date" class and the "timedelta" class from the "datetime" module.
- Next it calculates the date that occurred 5 days before the current date (date.today()) using the "timedelta" class. This is done by subtracting a "timedelta" object of 5 days from the current date.
- Finally it prints two lines:
- The current date is printed using "date.today()".
- The date calculated 5 days before the current date is printed using the previously calculated "dt".
Flowchart:
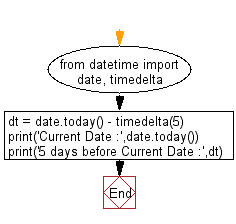
For more Practice: Solve these Related Problems:
- Write a Python program to subtract five days from a given date and then display the result in "DD/MM/YYYY" format.
- Write a Python script to compute the date five days prior to today and then check if that date falls on a weekend.
- Write a Python program that subtracts five days from the current date and then adds two weeks to the result, displaying both dates.
- Write a Python function to subtract a variable number of days (input by the user) from today's date and output the weekday of the resulting date.
Go to:
Previous: Write a Python program to get the current time.
Next: Write a Python program to convert unix timestamp string to readable date.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.