Python: Generate RFC 3339 timestamp
51. RFC 3339 Timestamp Generator
Write a Python program to generate RFC 3339 timestamp.
Sample Solution:
Python Code:
# Import the datetime and timezone classes from the datetime module
from datetime import datetime, timezone
# Get the current UTC time and convert it to the local timezone
local_time = datetime.now(timezone.utc).astimezone()
# Print an empty line for formatting purposes
print()
# Print the local time in ISO 8601 format
print(local_time.isoformat())
# Print an empty line for formatting purposes
print()
Output:
2017-05-08T17:30:34.009056+05:30
Explanation:
In the exercise above,
- The code imports the "datetime" and "timezone" classes from the "datetime" module.
- It gets the current time in UTC timezone using "datetime.now(timezone.utc)".
- It converts the UTC time to the local timezone using the "astimezone()" method.
- It prints the local time in ISO 8601 format using the "isoformat()" method, which represents the datetime object in a standardized format.
Flowchart:
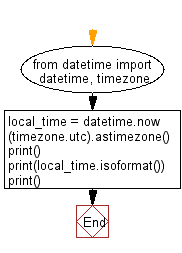
For more Practice: Solve these Related Problems:
- Write a Python program to generate the current timestamp in RFC 3339 format and then adjust it for a specified timezone.
- Write a Python script to convert a given datetime to an RFC 3339 formatted string and then verify its compliance with the standard.
- Write a Python function to generate RFC 3339 timestamps for a list of dates and then sort them.
- Write a Python program to output the current time as an RFC 3339 timestamp and then compare it with the ISO 8601 format.
Go to:
Previous: Write a Python program to get a list of dates between two dates.
Next: Write a Python program to get the first and last second.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.