Python: Get the time values with components using local time and gmtime
56. Time Components Fetcher
Write a Python program to get time values with components using local time and gmtime.
Sample Solution:
Python Code:
# Import the time module
import time
# Define a function named time_struct to print various attributes of a time structure
def time_struct(s):
# Print the year
print(' tm_year :', s.tm_year)
# Print the month
print(' tm_mon :', s.tm_mon)
# Print the day of the month
print(' tm_mday :', s.tm_mday)
# Print the hour
print(' tm_hour :', s.tm_hour)
# Print the minute
print(' tm_min :', s.tm_min)
# Print the second
print(' tm_sec :', s.tm_sec)
# Print the day of the week (0 for Monday, 6 for Sunday)
print(' tm_wday :', s.tm_wday)
# Print the day of the year (1 to 366)
print(' tm_yday :', s.tm_yday)
# Print whether daylight saving time is in effect (0, 1, or -1)
print(' tm_isdst:', s.tm_isdst)
# Print a message indicating local time
print('\nlocaltime:')
# Call the time_struct function with the current local time
time_struct(time.localtime())
# Print a message indicating UTC time
print('\ngmtime:')
# Call the time_struct function with the current UTC time
time_struct(time.gmtime())
Output:
localtime: tm_year : 2021 tm_mon : 4 tm_mday : 13 tm_hour : 11 tm_min : 20 tm_sec : 37 tm_wday : 1 tm_yday : 103 tm_isdst: 0 gmtime: tm_year : 2021 tm_mon : 4 tm_mday : 13 tm_hour : 11 tm_min : 20 tm_sec : 37 tm_wday : 1 tm_yday : 103 tm_isdst: 0
Explanation:
In the exercise above,
- The code imports the "time" module.
- It defines a function named "time_struct()" to print various attributes of a time structure.
- Inside the time_struct function:
- It prints the year, month, day of the month, hour, minute, second, day of the week (0 for Monday, 6 for Sunday), day of the year (1 to 366), and whether daylight saving time is in effect (0, 1, or -1) for the given time structure.
- It prints a message indicating local time.
- It calls the "time_struct()" function with the current local time obtained using "time.localtime()".
- It prints a message indicating UTC time.
- It calls the "time_struct()" function with the current UTC time obtained using "time.gmtime()".
Flowchart:
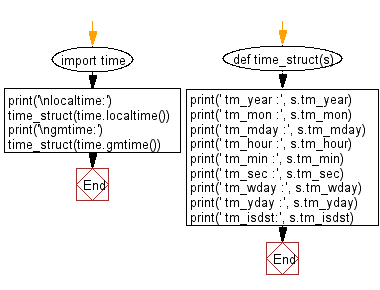
For more Practice: Solve these Related Problems:
- Write a Python program to display local time components (year, month, day, hour, minute, second) using time.localtime and then print them individually.
- Write a Python script to fetch the current local time structure and then output each component on a separate line.
- Write a Python function to extract and print the components of the current time using both localtime and gmtime for comparison.
- Write a Python program to get the current time in a structured format and then convert it into a formatted string showing all components.
Go to:
Previous: The epoch is the point where the time starts, and is platform dependent. For Unix, the epoch is January 1, 1970, 00:00:00 (UTC). Write a Python program to find out what the epoch is on a given platform. Also convert a given time in seconds since the epoch.
Next: Write a Python program to get different time values with components timezone, timezone abbreviations, the offset of the local (non-DST) timezone, DST timezone and time of different timezones.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.