Python: Get different time values with components timezone, timezone abbreviations
57. Timezone Components Fetcher
Write a Python program to get different time values with components timezone, timezone abbreviations, the offset of the local (non-DST) timezone, DST timezone and time of different timezones.
Sample Solution:
Python Code:
# Import the time module
import time
# Import the os module
import os
# Define a function named zone_info to print timezone information
def zone_info():
# Print the current value of the TZ environment variable
print('TZ :', os.environ.get('TZ', '(not set)'))
# Print the timezone abbreviations (Eastern, Pacific, etc.)
print('Timezone abbreviations:', time.tzname)
# Print the timezone offset from UTC in seconds, and in hours
print('Timezone : {} ({})'.format(
time.timezone, (time.timezone / 3600)))
# Print whether daylight saving time (DST) is in effect
print('DST timezone ', time.daylight)
# Print the current time with formatting (%X: time, %x: date, %Z: timezone)
print('Time :', time.strftime('%X %x %Z'),'\n')
# Print a message indicating default timezone
print('Default Zone:')
# Call the zone_info function to print information about the default timezone
zone_info()
# Define a list of timezones to be tested
TIME_ZONES = [
'Pacific/Auckland',
'Europe/Berlin',
'America/Detroit',
'Singapore',
]
# Iterate over each timezone in the TIME_ZONES list
for zone in TIME_ZONES:
# Set the TZ environment variable to the current timezone
os.environ['TZ'] = zone
# Apply the timezone change
time.tzset()
# Print the current timezone
print(zone, ':')
# Call the zone_info function to print information about the current timezone
zone_info()
Output:
Default Zone: TZ : (not set) Timezone abbreviations: ('UTC', 'UTC') Timezone : 0 (0.0) DST timezone 0 Time : 11:30:05 04/13/21 UTC Pacific/Auckland : TZ : Pacific/Auckland Timezone abbreviations: ('NZST', 'NZDT') Timezone : -43200 (-12.0) DST timezone 1 Time : 23:30:05 04/13/21 NZST Europe/Berlin : TZ : Europe/Berlin Timezone abbreviations: ('CET', 'CEST') Timezone : -3600 (-1.0) DST timezone 1 Time : 13:30:05 04/13/21 CEST America/Detroit : TZ : America/Detroit Timezone abbreviations: ('EST', 'EDT') Timezone : 18000 (5.0) DST timezone 1 Time : 07:30:05 04/13/21 EDT Singapore : TZ : Singapore Timezone abbreviations: ('+08', '+08') Timezone : -28800 (-8.0) DST timezone 0 Time : 19:30:05 04/13/21 +08
Explanation:
In the exercise above,
- The code imports the "time" and "os" modules.
- It defines a function named "zone_info()" to print timezone information, such as the value of the "TZ" environment variable, timezone abbreviations, timezone offset from UTC, and whether daylight saving time (DST) is in effect.
- It prints a message indicating the default timezone and calls the "zone_info()" function to print information about it.
- It defines a list of timezones ('TIME_ZONES') to be tested.
- It iterates over each timezone in the 'TIME_ZONES' list.
- Inside the loop:
- It sets the 'TZ' environment variable to the current timezone.
- It applies the timezone change using "time.tzset()".
- It prints the name of the current timezone.
- It calls the "zone_info()" function to print information about the current timezone.
Flowchart:
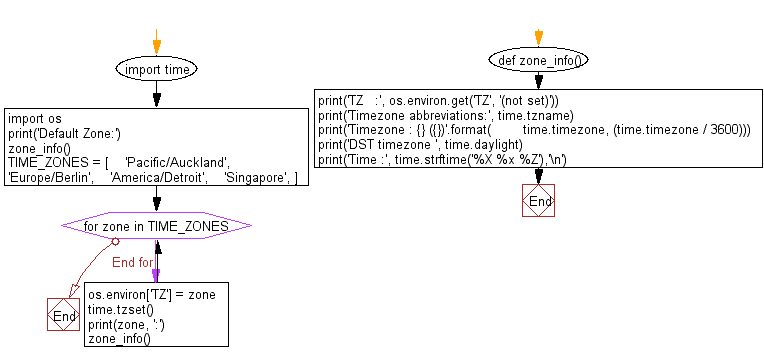
For more Practice: Solve these Related Problems:
- Write a Python program to display detailed timezone information including timezone name, offset, and DST status using the time module.
- Write a Python script to fetch and print the local timezone, its abbreviation, and offset from UTC for both standard and daylight saving times.
- Write a Python function to retrieve and compare timezone components for multiple timezones (e.g., UTC, EST, PST) and then display the differences.
- Write a Python program to get the current local time along with its timezone information and then format the output to include both local and DST details.
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to get time values with components using local time and gmtime.
Next: Write a Python program that can suspend execution of a given script a given number of seconds.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.