Python: Suspend execution of a given script a given number of seconds
Python Datetime: Exercise-58 with Solution
Write a Python program that can suspend execution of a given script for a given number of seconds.
Sample Solution:
Python Code:
# Import the time module
import time
# Iterate over a range of 4 values
for x in range(4):
# Pause the execution for 3 seconds
time.sleep(3)
# Print a message indicating that the program slept for 3 seconds
print("Sorry, Slept for 3 seconds...")
Output:
Sorry, Slept for 3 seconds... Sorry, Slept for 3 seconds... Sorry, Slept for 3 seconds... Sorry, Slept for 3 seconds...
Explanation:
In the exercise above,
- The code imports the "time" module, which provides functions for working with time.
- It iterates over a range of 4 values using a "for" loop.
- Inside the loop:
- It pauses the execution of the program for 3 seconds using the "time.sleep(3)" function call.
- It prints a message indicating that the program has slept for 3 seconds. This message will be printed 4 times, as the loop iterates 4 times.
Flowchart:
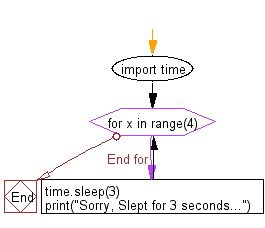
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to get different time values with components timezone, timezone abbreviations, the offset of the local (non-DST) timezone, DST timezone and time of different timezones.
Next: Write a Python program to convert a given time in seconds since the epoch to a string representing local time.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/date-time-exercise/python-date-time-exercise-58.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics