Python: Convert unix timestamp string to readable date
Python datetime: Exercise-6 with Solution
Write a Python program to convert a Unix timestamp string to a readable date.
Sample Solution:
Python Code:
# Import the datetime module
import datetime
# Convert the given timestamp (as a string) to an integer and then convert it to a datetime object
# The timestamp '1284105682' represents the number of seconds since the epoch (January 1, 1970)
# Convert the datetime object to a string representation using the specified format '%Y-%m-%d %H:%M:%S'
# and print it
print(
datetime.datetime.fromtimestamp(
int("1284105682")
).strftime('%Y-%m-%d %H:%M:%S')
)
Output:
2010-09-10 13:31:22
Explanation:
In the exercise above,
- The code imports the "datetime" module, which provides functionalities to work with dates and times.
- Converting Timestamp:
- It converts the given timestamp string "1284105682" into an integer using the "int()" function.
- Then, it uses datetime.datetime.fromtimestamp() method to convert the integer timestamp into a datetime object. This method creates a datetime object representing the specified timestamp, where the timestamp represents the number of seconds since the epoch (January 1, 1970).
- After converting the timestamp into a datetime object, it formats the datetime object into a string representation using the "strftime()" method with the specified format '%Y-%m-%d %H:%M:%S'. This format represents the datetime as "Year-Month-Day Hour:Minute:Second".
- Finally, it prints the formatted datetime string.
Flowchart:
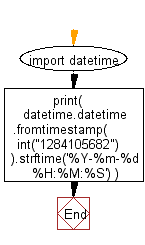
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to subtract five days from current date.
Next: Write a Python program to print yesterday, today, tomorrow.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/date-time-exercise/python-date-time-exercise-6.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics