Python: A tuple containing 9 elements corresponding to structure time as an argument and returns a string representing it
62. Time Tuple to String
Write a Python program that takes a tuple containing 9 elements corresponding to structure time as an argument and returns a string representing it.
Sample Solution:
Python Code:
# Import the time module
import time
# Define a tuple 't' representing a specific time: (year, month, day, hour, minute, second, day of the week, day of the year, daylight saving time)
t = (2020, 1, 22, 2, 34, 6, 6, 362, 0)
# Convert the given time tuple 't' into a string representing the local time and store it in the variable 'result'
result = time.asctime(t)
# Print a message indicating the result of the conversion
print("Result:", result)
# Define another tuple 't' representing a different specific time: (year, month, day, hour, minute, second, day of the week, day of the year, daylight saving time)
t = (1982, 11, 12, 2, 54, 8, 8, 360, 0)
# Convert the given time tuple 't' into a string representing the local time and store it in the variable 'result'
result = time.asctime(t)
# Print a message indicating the result of the conversion
print("Result:", result)
Output:
Result: Sun Jan 22 02:34:06 2020 Result: Tue Nov 12 02:54:08 1982
Explanation:
- The code imports the "time" module, which provides functions for working with time.
- It defines a tuple 't' representing a specific time with various components such as year, month, day, hour, minute, second, etc.
- It uses the "time.asctime()" function to convert the given time tuple 't' into a string representing local time.
- It stores the resulting string in the variable named 'result'.
- It prints a message indicating the result of the conversion.
- It repeats the process for another tuple 't' representing a different specific time and prints the result of the conversion for that time as well.
Flowchart:
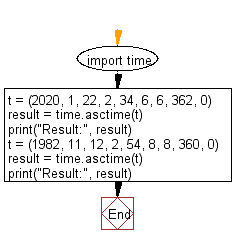
For more Practice: Solve these Related Problems:
- Write a Python program that takes a tuple representing time.struct_time and returns a formatted date string.
- Write a Python script to convert a 9-element time tuple into a human-readable string using time.strftime.
- Write a Python function to accept a time tuple and then output the corresponding time in "Day Mon DD HH:MM:SS YYYY" format.
- Write a Python program to transform a time.struct_time tuple into a custom formatted string and then print the result.
Go to:
Previous: Write a Python program that takes a given number of seconds and pass since epoch as an argument. Print structure time in local time.
Next: Write a Python program to parse a string representing time and returns the structure time.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.