Python: Parse a string representing time and returns the structure time
63. Parse Time String
Write a Python program to parse a string representing time and return the time structure.
Sample Solution:
Python Code:
# Import the time module
import time
# Define a string representing a time
time_string = "22 January, 2020"
# Print a message indicating the string representing time
print("String representing time:", time_string)
# Parse the time string using the specified format and store the result in the variable 'result'
result = time.strptime(time_string, "%d %B, %Y")
# Print the result
print(result)
# Define another string representing a time
time_string = "30 Nov 00"
# Print a message indicating the string representing time
print("\nString representing time:", time_string)
# Parse the time string using the specified format and store the result in the variable 'result'
result = time.strptime(time_string, "%d %b %y")
# Print the result
print(result)
# Define another string representing a time
time_string = '04/11/15 11:55:23'
# Print a message indicating the string representing time
print("\nString representing time:", time_string)
# Parse the time string using the specified format and store the result in the variable 'result'
result = time.strptime(time_string, "%m/%d/%y %H:%M:%S")
# Print the result
print(result)
# Define another string representing a time
time_string = '12-11-2019'
# Print a message indicating the string representing time
print("\nString representing time:", time_string)
# Parse the time string using the specified format and store the result in the variable 'result'
result = time.strptime(time_string, "%m-%d-%Y")
# Print the result
print(result)
# Define another string representing a time
time_string = '13::55::26'
# Print a message indicating the string representing time
print("\nString representing time:", time_string)
# Parse the time string using the specified format and store the result in the variable 'result'
result = time.strptime(time_string, "%H::%M::%S")
# Print the result
print(result)
Output:
String representing time: 22 January, 2020 time.struct_time(tm_year=2020, tm_mon=1, tm_mday=22, tm_hour=0, tm_min=0, tm_sec=0, tm_wday=2, tm_yday=22, tm_isdst=-1) String representing time: 30 Nov 00 time.struct_time(tm_year=2000, tm_mon=11, tm_mday=30, tm_hour=0, tm_min=0, tm_sec=0, tm_wday=3, tm_yday=335, tm_isdst=-1) String representing time: 04/11/15 11:55:23 time.struct_time(tm_year=2015, tm_mon=4, tm_mday=11, tm_hour=11, tm_min=55, tm_sec=23, tm_wday=5, tm_yday=101, tm_isdst=-1) String representing time: 12-11-2019 time.struct_time(tm_year=2019, tm_mon=12, tm_mday=11, tm_hour=0, tm_min=0, tm_sec=0, tm_wday=2, tm_yday=345, tm_isdst=-1) String representing time: 13::55::26 time.struct_time(tm_year=1900, tm_mon=1, tm_mday=1, tm_hour=13, tm_min=55, tm_sec=26, tm_wday=0, tm_yday=1, tm_isdst=-1)
Explanation:
In the exercise above,
- The code imports the "time" module, which provides functions for working with time.
- It defines several strings representing different formats of time.
- It prints each string representing the time before parsing.
- It uses the "time.strptime()" function to parse each time string using the specified format.
- It prints the 'result' of each parsing operation. The 'result' is a time tuple containing various components such as year, month, day, hour, minute, second, etc., depending on the format of the input string.
Flowchart:
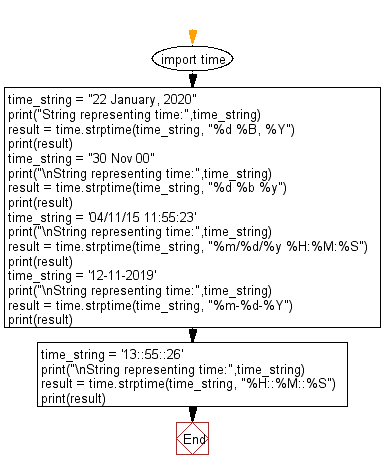
For more Practice: Solve these Related Problems:
- Write a Python program to parse various time strings into time.struct_time objects using time.strptime and then display each component.
- Write a Python script that takes a time string in a non-standard format and returns the parsed time structure.
- Write a Python function to convert multiple time strings into struct_time and then verify the conversion by reformatting them.
- Write a Python program to parse a time string with ambiguous delimiters and then output the corresponding time.struct_time with error handling.
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program that takes a tuple containing 9 elements corresponding to structure time as an argument and returns a string representing it.
Next: Python Class exercises
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.