Python: Print yesterday, today, tomorrow
Write a Python program to print yesterday, today, tomorrow.
Sample Solution:
Python Code:
# Import the datetime module
import datetime
# Get the current date using datetime.date.today()
today = datetime.date.today()
# Calculate yesterday's date by subtracting 1 day from today's date using timedelta(days=1)
yesterday = today - datetime.timedelta(days=1)
# Calculate tomorrow's date by adding 1 day to today's date using timedelta(days=1)
tomorrow = today + datetime.timedelta(days=1)
# Print yesterday's date
print('Yesterday : ', yesterday)
# Print today's date
print('Today : ', today)
# Print tomorrow's date
print('Tomorrow : ', tomorrow)
Output:
Yesterday : 2017-05-05 Today : 2017-05-06 Tomorrow : 2017-05-07
Explanation:
In the exercise above,
- The code imports the "datetime" module, which provides functionalities to work with dates and times.
- Get Current Date:
- It retrieves the current date using the "datetime.date.today()" method and assigns it to the variable 'today'.
- Calculating Previous and Next Dates:
- It calculates yesterday's date by subtracting one day from the current date ('today') using the "datetime.timedelta(days=1)" function. The result is assigned to the variable 'yesterday'.
- It calculates tomorrow's date by adding one day to the current date ('today') using the "datetime.timedelta(days=1)" function. The result is assigned to the variable 'tomorrow'.
- Finally it prints the calculated dates ('yesterday', 'today', and 'tomorrow') along with their corresponding labels.
Flowchart:
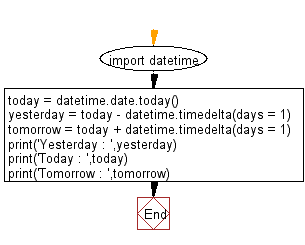
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to convert unix timestamp string to readable date.
Next: Write a Python program to convert the date to datetime (midnight of the date).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics