Python: Convert the date to datetime (midnight of the date)
Python datetime: Exercise-8 with Solution
Write a Python program to convert the date to datetime (midnight of the date) in Python.
Sample Solution:
Python Code:
# Import the date class from the datetime module
from datetime import date
# Import the datetime class from the datetime module
from datetime import datetime
# Get the current date and assign it to the variable 'dt'
dt = date.today()
# Combine the current date 'dt' with the minimum time value (00:00:00) of a datetime object
# This effectively creates a datetime object with the date component from 'dt' and the time component as the minimum possible time
# Print the resulting datetime object
print(datetime.combine(dt, datetime.min.time()))
Output:
2017-05-06 00:00:00
Explanation:
In the exercise above,
- The code imports the "date" class and the "datetime" class from the "datetime" module.
- Get Current Date:
- It retrieves the current date using the "date.today()" method and assigns it to the variable "dt".
- Combining Date and Time:
- It combines the current date "dt" with the minimum possible time value (00:00:00) of a datetime object using the "datetime.combine()" method.
- This effectively creates a datetime object with the date component from "dt" and the time component as the minimum possible time.
- Finally it prints the resulting datetime object, which represents the current date with the time component set to the minimum possible time (midnight).
Flowchart:
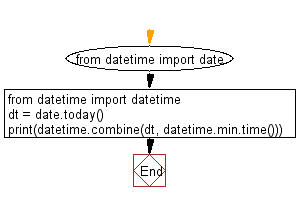
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to print yesterday, today, tomorrow.
Next: Write a Python program to print next 5 days starting from today.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/date-time-exercise/python-date-time-exercise-8.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics