Implementing a Python decorator for argument validation
5. Implement a Decorator to Validate Function Arguments
Write a Python program that implements a decorator to validate function arguments based on a given condition.
Sample Solution:
Python Code:
def validate_arguments(condition):
def decorator(func):
def wrapper(*args, **kwargs):
if condition(*args, **kwargs):
return func(*args, **kwargs)
else:
raise ValueError("Invalid arguments passed to the function")
return wrapper
return decorator
@validate_arguments(lambda x: x > 0)
def calculate_cube(x):
return x ** 3
print(calculate_cube(5)) # Output: 125
print(calculate_cube(-2)) # Raises ValueError: Invalid arguments passed to the function
Sample Output:
125 Traceback (most recent call last): ......... print(calculate_cube(-2)) # Raises ValueError: Invalid arguments passed to the function ........................ raise ValueError("Invalid arguments passed to the function") ValueError: Invalid arguments passed to the function
Explanation:
In the above exercise, the "calculate_cube()" function is decorated with @validate_arguments. The validation condition is specified as a lambda function lambda x: x > 0, which checks if the argument x is greater than 0. When "calculate_cube()" is called with a positive number, it executes normally and returns the square of the input. However, if it's called with a negative number, it raises a ValueError indicating invalid arguments were passed.
Flowchart:
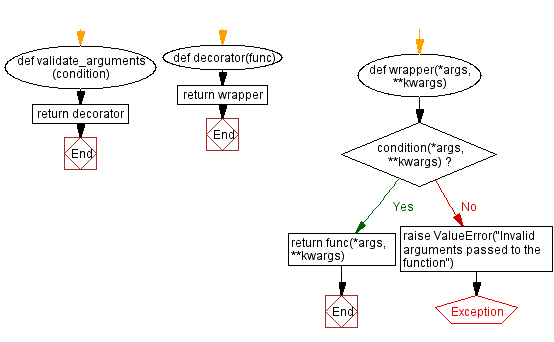
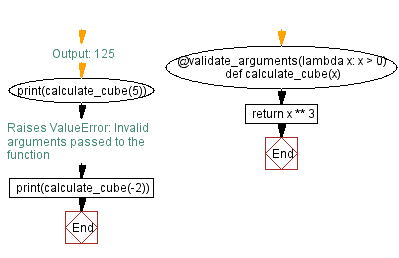
For more Practice: Solve these Related Problems:
- Write a Python decorator that checks if all arguments passed to a function are positive integers and raises a ValueError otherwise.
- Write a Python decorator that uses a user-defined predicate function to validate the input arguments before function execution.
- Write a Python decorator that verifies that the number of arguments passed to a function is within a specified range.
- Write a Python decorator that asserts the type of each argument, and if any mismatch occurs, logs the error and raises a TypeError.
Go to:
Previous: Implementing a Python decorator for function results caching.
Next: Implementing a Python decorator for function retry.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.