Implementing a Python decorator for exception handling with a default response
Python Decorator: Exercise-9 with Solution
Write a Python program that implements a decorator to handle exceptions raised by a function and provide a default response.
Sample Solution:
Python Code:
def handle_exceptions(default_response):
def decorator(func):
def wrapper(*args, **kwargs):
try:
# Call the original function
return func(*args, **kwargs)
except Exception as e:
# Handle the exception and provide the default response
print(f"Exception occurred: {e}")
return default_response
return wrapper
return decorator
# Example usage
@handle_exceptions(default_response="An error occurred!")
def divide_numbers(x, y):
return x / y
# Call the decorated function
result = divide_numbers(7, 0) # This will raise a ZeroDivisionError
print("Result:", result)
Sample Output:
Exception occurred: division by zero Result: An error occurred
Explanation:
In the above exercise -
The 'handle_exceptions' decorator takes a default response as an argument. It then defines a decorator function that wraps around the original function. Within the wrapper function, the original function is called within a try-except block. In the case of an exception during the execution of the original function, the default response is returned instead.
In the example usage, the 'divide_numbers' function is decorated with @handle_exceptions(default_response="An error occurred"). When 'divide_numbers' is called with '7' and '0', a 'ZeroDivisionError' will be raised. However, the decorator catches the exception, prints an error message, and returns the default response "An error occurred".
Flowchart:
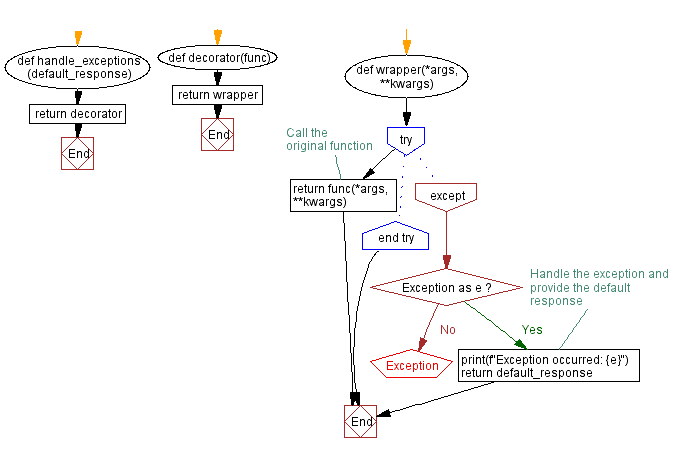
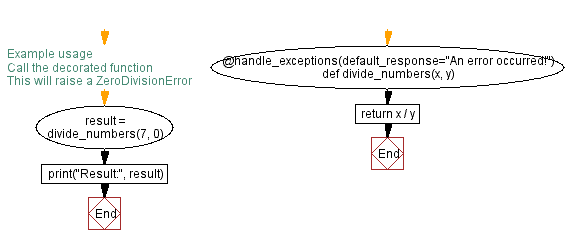
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Implementing a Python decorator for function logging.
Next: Implementing a Python decorator for enforcing type checking on function arguments.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/decorator/python-decorator-exercise-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics