Python: Sort (ascending and descending) a dictionary by value
1. Sort Dictionary by Value
Write a Python program to sort (ascending and descending) a dictionary by value.
Sample Solution-1:
Python Code:
# Import the 'operator' module, which provides functions for common operations like sorting.
import operator
# Create a dictionary 'd' with key-value pairs.
d = {1: 2, 3: 4, 4: 3, 2: 1, 0: 0}
# Print the original dictionary 'd'.
print('Original dictionary : ',d)
# Sort the items (key-value pairs) in the dictionary 'd' based on the values (1st element of each pair).
# The result is a list of sorted key-value pairs.
sorted_d = sorted(d.items(), key=operator.itemgetter(1))
# Print the dictionary 'sorted_d' in ascending order by value.
print('Dictionary in ascending order by value : ',sorted_d)
# Convert the sorted list of key-value pairs back into a dictionary.
# The 'reverse=True' argument sorts the list in descending order by value.
sorted_d = dict( sorted(d.items(), key=operator.itemgetter(1), reverse=True))
# Print the dictionary 'sorted_d' in descending order by value.
print('Dictionary in descending order by value : ',sorted_d)
Sample Output:
Original dictionary : {1: 2, 3: 4, 4: 3, 2: 1, 0: 0} Dictionary in ascending order by value : [(0, 0), (2, 1), (1, 2), (4, 3), (3, 4)] Dictionary in descending order by value : {3: 4, 4: 3, 1: 2, 2: 1, 0: 0}
Sample Solution-2:
Note: Dictionary values must be of the same type.
- Use dict.items() to get a list of tuple pairs from d and sort it using a lambda function and sorted().
- Use dict() to convert the sorted list back to a dictionary.
- Use the reverse parameter in sorted() to sort the dictionary in reverse order, based on the second argument.
Python Code:
# Define a function 'sort_dict_by_value' that takes a dictionary 'd' and an optional 'reverse' flag.
# It returns the dictionary sorted by values in ascending or descending order, based on the 'reverse' flag.
def sort_dict_by_value(d, reverse = False):
return dict(sorted(d.items(), key = lambda x: x[1], reverse = reverse))
# Print a message indicating the start of the code section.
print("Original dictionary elements:")
# Create a dictionary 'colors' with key-value pairs.
colors = {'Red': 1, 'Green': 3, 'Black': 5, 'White': 2, 'Pink': 4}
# Print the original dictionary 'colors'.
print(colors)
# Print a message indicating the start of the sorting process in ascending order.
print("\nSort (ascending) the said dictionary elements by value:")
# Call the 'sort_dict_by_value' function to sort the 'colors' dictionary by value in ascending order.
# Print the result.
print(sort_dict_by_value(colors))
# Print a message indicating the start of the sorting process in descending order.
print("\nSort (descending) the said dictionary elements by value:")
# Call the 'sort_dict_by_value' function with 'reverse=True' to sort the 'colors' dictionary by value in descending order.
# Print the result.
print(sort_dict_by_value(colors, True))
Sample Output:
Original dictionary elements: {'Red': 1, 'Green': 3, 'Black': 5, 'White': 2, 'Pink': 4} Sort (ascending) the said dictionary elements by value: {'Red': 1, 'White': 2, 'Green': 3, 'Pink': 4, 'Black': 5} Sort (descending) the said dictionary elements by value: {'Black': 5, 'Pink': 4, 'Green': 3, 'White': 2, 'Red': 1}
Flowchart:
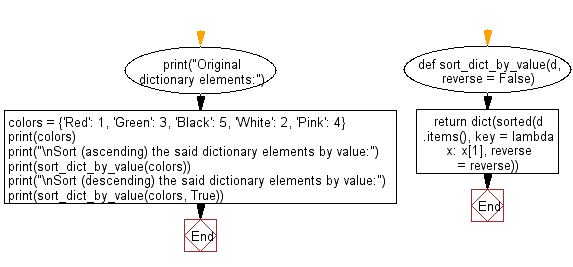
For more Practice: Solve these Related Problems:
- Write a Python script to sort a dictionary by its values in ascending order using lambda functions.
- Write a Python script to sort a dictionary by its values in descending order and output the sorted key-value pairs as tuples.
- Write a Python script to implement dictionary sorting by value without using the built‐in sorted() function.
- Write a Python script to compare two different methods of sorting a dictionary by value and print their results.
Go to:
Previous: Python Dictionary Exercise Home.
Next: Write a Python program to add a key to a dictionary.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.