Python: Remove a key from a dictionary
Python dictionary: Exercise-12 with Solution
Write a Python program to remove a key from a dictionary.
Visual Presentation:
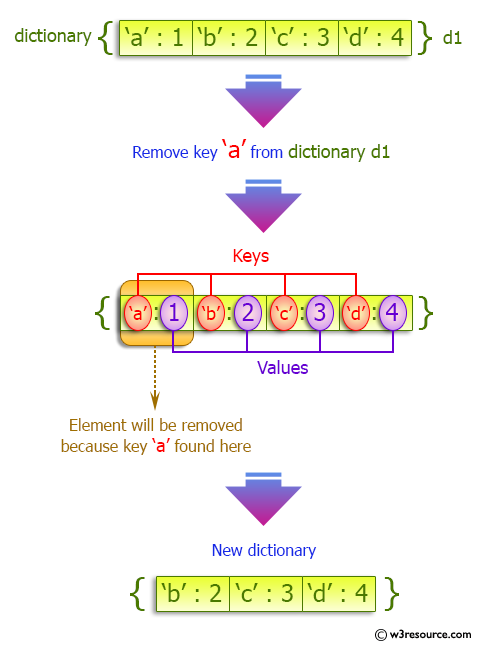
Sample Solution:
Python Code:
# Create a dictionary 'myDict' with key-value pairs.
myDict = {'a': 1, 'b': 2, 'c': 3, 'd': 4}
# Print the original dictionary 'myDict'.
print(myDict)
# Check if the key 'a' exists in the 'myDict' dictionary.
if 'a' in myDict:
# If 'a' is in the dictionary, delete the key-value pair with the key 'a'.
del myDict['a']
# Print the updated dictionary 'myDict' after deleting the key 'a' (if it existed).
print(myDict)
Sample Output:
{'a': 1, 'b': 2, 'c': 3, 'd': 4} {'b': 2, 'c': 3, 'd': 4}
Python Code Editor:
Previous: Write a Python program to multiply all the items in a dictionary.
Next: Write a Python program to map two lists into a dictionary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/dictionary/python-data-type-dictionary-exercise-12.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics