Python: Remove spaces from dictionary keys
29. Remove Spaces from Dictionary Keys
Write a Python program to remove spaces from dictionary keys.
Visual Presentation:
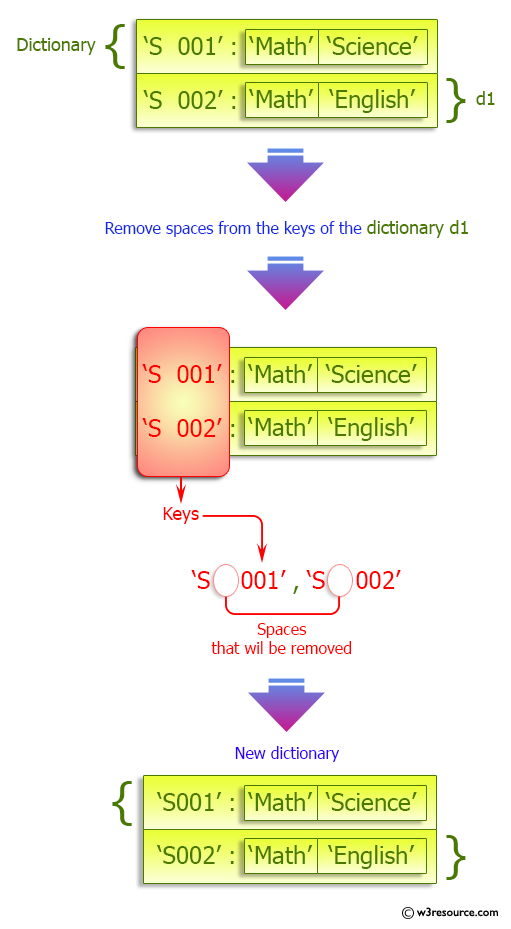
Sample Solution:
Python Code:
# Create a dictionary 'student_list' with keys containing extra spaces and associated lists of subjects.
student_list = {'S 001': ['Math', 'Science'], 'S 002': ['Math', 'English']}
# Print a message indicating the start of the code section.
print("Original dictionary: ", student_list)
# Use a dictionary comprehension to create a new dictionary 'student_dict'.
# Iterate through the key-value pairs in 'student_list'.
# Remove extra spaces from the keys using the 'translate' method with a translation table.
student_dict = {x.translate({32: None}): y for x, y in student_list.items()}
# Print a message indicating the start of the new dictionary section.
print("New dictionary: ", student_dict)
Sample Output:
Original dictionary: {'S 001': ['Math', 'Science'], 'S 002': ['Math', 'English']} New dictionary: {'S001': ['Math', 'Science'], 'S002': ['Math', 'English']}
For more Practice: Solve these Related Problems:
- Write a Python program to remove all spaces from the keys of a dictionary and return a new dictionary.
- Write a Python program to iterate over a dictionary and strip whitespace from each key.
- Write a Python program to use dictionary comprehension to create a dictionary with keys that have no spaces.
- Write a Python program to implement a function that cleans dictionary keys by removing spaces and special characters.
Go to:
Previous: Write a Python program to sort a list alphabetically in a dictionary.
Next: Write a Python program to get the top three items in a shop.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.