Python: Concatenate following dictionaries to create a new one
Python dictionary: Exercise-3 with Solution
Write a Python script to concatenate the following dictionaries to create a new one.
Visual Presentation:
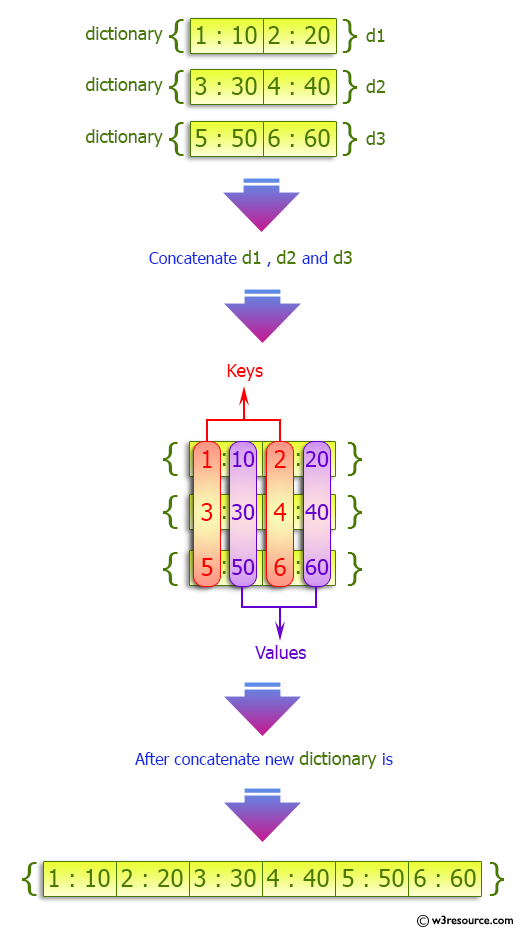
Sample Solution:
Python Code:
# Create three dictionaries 'dic1', 'dic2', and 'dic3' with key-value pairs.
dic1 = {1: 10, 2: 20}
dic2 = {3: 30, 4: 40}
dic3 = {5: 50, 6: 60}
# Create an empty dictionary 'dic4' that will store the combined key-value pairs from 'dic1', 'dic2', and 'dic3'.
dic4 = {}
# Iterate through each dictionary ('dic1', 'dic2', and 'dic3') using a loop.
for d in (dic1, dic2, dic3):
# Update 'dic4' by adding the key-value pairs from the current dictionary 'd'.
dic4.update(d)
# Print the combined dictionary 'dic4' containing all the key-value pairs from 'dic1', 'dic2', and 'dic3'.
print(dic4)
Sample Output:
{1: 10, 2: 20, 3: 30, 4: 40, 5: 50, 6: 60}
Python Code Editor:
Previous: Write a Python program to add a key to a dictionary.
Next: Write a Python program to check if a given key already exists in a dictionary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/dictionary/python-data-type-dictionary-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics