Python: Get the top three items in a shop
30. Get Top Three Items in a Shop
Write a Python program to get the top three items in a shop.
Visual Presentation:
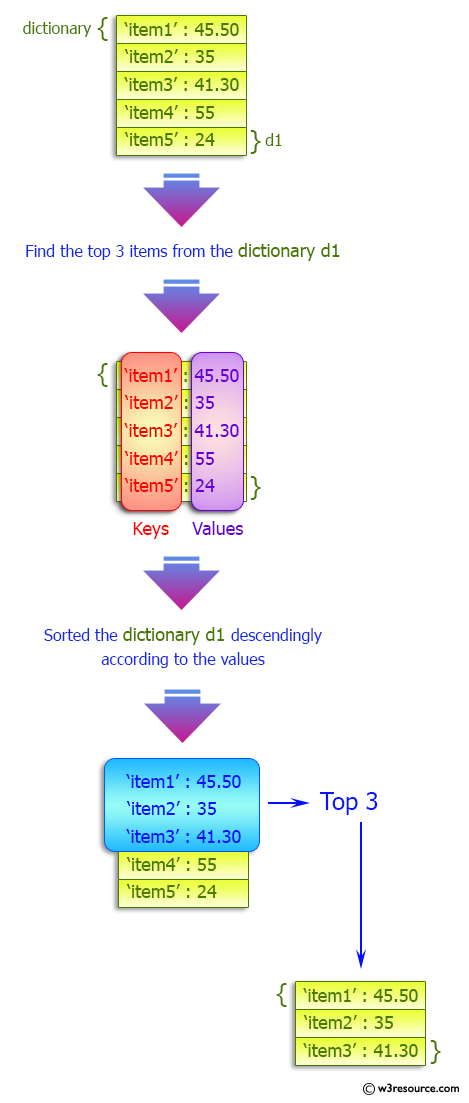
Sample Solution:
Python Code:
# Import the 'nlargest' function from the 'heapq' module and the 'itemgetter' function from the 'operator' module.
from heapq import nlargest
from operator import itemgetter
# Create a dictionary 'items' with keys representing items and values as their corresponding prices.
items = {'item1': 45.50, 'item2': 35, 'item3': 41.30, 'item4': 55, 'item5': 24}
# Use the 'nlargest' function to find the three items with the largest prices from the 'items' dictionary.
# The 'key' argument specifies that the second element (price) of each key-value pair should be used for comparison.
for name, value in nlargest(3, items.items(), key=itemgetter(1)):
# Print the name and price of the three items with the largest prices.
print(name, value)
Sample Output:
item4 55 item1 45.5 item3 41.3
For more Practice: Solve these Related Problems:
- Write a Python program to sort a dictionary of shop items by price in descending order and print the top three.
- Write a Python program to use heapq.nlargest to extract the top three most expensive items from a dictionary.
- Write a Python program to implement a function that returns the three highest-priced items from a shop inventory dictionary.
- Write a Python program to iterate over a shop dictionary and output the top three key-value pairs based on value.
Go to:
Previous: Write a Python program to remove spaces from dictionary keys.
Next: Write a Python program to get the key, value and item in a dictionary.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.